HTTP request parameter acquisition, cookie setting and file upload
Access Request Instance
To get the current HTTP request instance in the controller, you need to set the Illuminate\Http\Request
Class, so that the current request instance will be automatically injected by the service container: <? php namespace App\Http\Controllers; use Illuminate\Http\Request; class UserController extends Controller { /** *Store new users * * @param Request $request * @return Response */ public function store(Request $request) { $name = $request->input('name'); // } }
Dependency Injection&Routing Parameters
If you also want to get the route parameters in the controller method, you just need to place the route parameters behind other dependencies. For example, if your route is defined as follows:
Route::put('user/{id}',' UserController@update ');
You can still Illuminate\Http\Request
Perform dependency injection and define controller methods to access routing parameters in the following way id
:
<? php namespace App\Http\Controllers; use Illuminate\Http\Request; class UserController extends Controller { /** *Update specified user * * @param Request $request * @param int $id * @return Response */ public function update(Request $request, $id) { // } }
Access requests through routing closures
You can also inject Illuminate\Http\Request
, the service container will automatically inject input requests when executing the closure function:
use Illuminate\Http\Request; Route::get('/', function (Request $request) { // });
Request Path&Method
Illuminate\Http\Request
Inherited from Symfony\Component\HttpFoundation\Request
Class, which provides multiple methods to detect the HTTP request of an application. Let's demonstrate some of the methods it provides to obtain the request path and request method: Get request path
path
Method will return the requested path information, so if the request URL is http://domain.com/user/1
, then path
Method will return user/1
:
$path = $request->path();
is
Method allows you to verify that the request path matches a given pattern. This method parameter supports *
Wildcard characters:
if($request->is('user/*')){ // }
If the request URL is http://domain.com/user/1
, this method will return true
。
Get Request URL
To get the complete URL, not just the path information, you can use the url
or fullUrl
method, url
The method returns a URL without a query string, while the fullUrl method returns a result containing a query string:
//Does not contain a query string $url = $request->url(); //Include query string $url_with_query = $request->fullUrl();
For example, we request http://domain.com/user/1?token=laravelacademy.org
, then the above $url
The value of is http://domain.com/user/1
, $url_with_query
The value of is http://blog.dev/user/1?token=laravelacademy.org
。
Get Request Method
method
Method will return the HTTP request mode. You can also use isMethod
Method to verify whether the HTTP request mode matches the given string:
$method = $request->method(); // GET/POST if($request->isMethod('post')){ // true or false }
PSR-7 request
PSR-7 standard Specifies the HTTP message interface, including request and response. If you want to obtain a request instance that complies with the PSR-7 standard instead of a Larravel request instance, you need to install some libraries first. Larravel can use Symfony HTTP Message Bridge The component converts the typical Laravel request and response into an implementation compatible with the PSR-7 interface: composer require symfony/psr-http-message-bridge composer require zendframework/zend-diactoros
After installing these libraries, you only need to prompt the type of request examples in the route or controller to obtain PSR-7 requests: use Psr\Http\Message\ServerRequestInterface; Route::get('/', function (ServerRequestInterface $request) { // });
By comparing the data structures of the Request instance and the ServerRequestInterface instance, we can see that the request instance of Laravel provides more information:


Note: If a PSR-7 response instance is returned from the route or controller, it will be automatically converted to a Laravel response instance and displayed.
Request string processing
By default, Larave App\Http\Kernel
Introduced in the global middleware stack of TrimStrings
and ConvertEmptyStringsToNull
Middleware. These middleware will automatically process the string fields in the request. The former will clear the spaces at both ends of the string, and the latter will convert the empty string into null
。 In this way, we do not need to do additional processing on string fields in the routing and controller:

If you want to prohibit this behavior, you can go from App\Http\Kernel
Middleware stack properties for $middleware
Remove these two middleware from.
Get request input
Get all input values
You can use all
Method to obtain all input values in array format:
$input = $request->all();
If the request URL is http://blog.dev/user/1?token=laravelacademy.org&name= Academician
, then corresponding $input
The return value is:

Get a single input value
Through some simple methods, you can Illuminate\Http\Request
Access user input in the instance. You don't need to care about the HTTP request method used for the request, because all request methods are input
Method to obtain user input:
$name = $request->input('name');
Take the above request URL as an example $name
Value is Academician
。
You can also pass a default value as the second parameter to input
Method, if the request input value does not appear in the current request URL, the value will be returned:
$name=$request ->input ('name ',' Scholar ');
For example, we visit http://blog.dev/user/1?token=laravelacademy.org
, you can still get $name
The value of is Academician
。
When processing form array input, you can use "." To access array input:
$input = $request->input('products.0.name'); $names = $request->input('products.*.name');
For example, we visit http://blog.dev/user/1?products [] [name]=Xueyuan Jun&products [] [name]=Xueyuan Jun trumpet
, then the above $input
The value of is Academician
, and $names
The value of is:

Get input from query string
input
Method will get the value from the entire request payload (including the query string), query
Then only the numeric value will be obtained from the query string:
$name = $request->query('name');
If no given query item is provided in the requested query string, we can input
In the same way, set the second parameter as the default value:
$name=$request ->query ('name ',' Scholar ');
You can also call a query
Method to obtain the value of the entire query string in an associative array, similar to all
What the method does:
$query = $request->query();
It is estimated that some people will be hoodwinked input
and query
What's the difference between them? The official documents are still a little ambiguous. So here, we will talk about the essential difference between the two and go back to print the picture of the request instance:
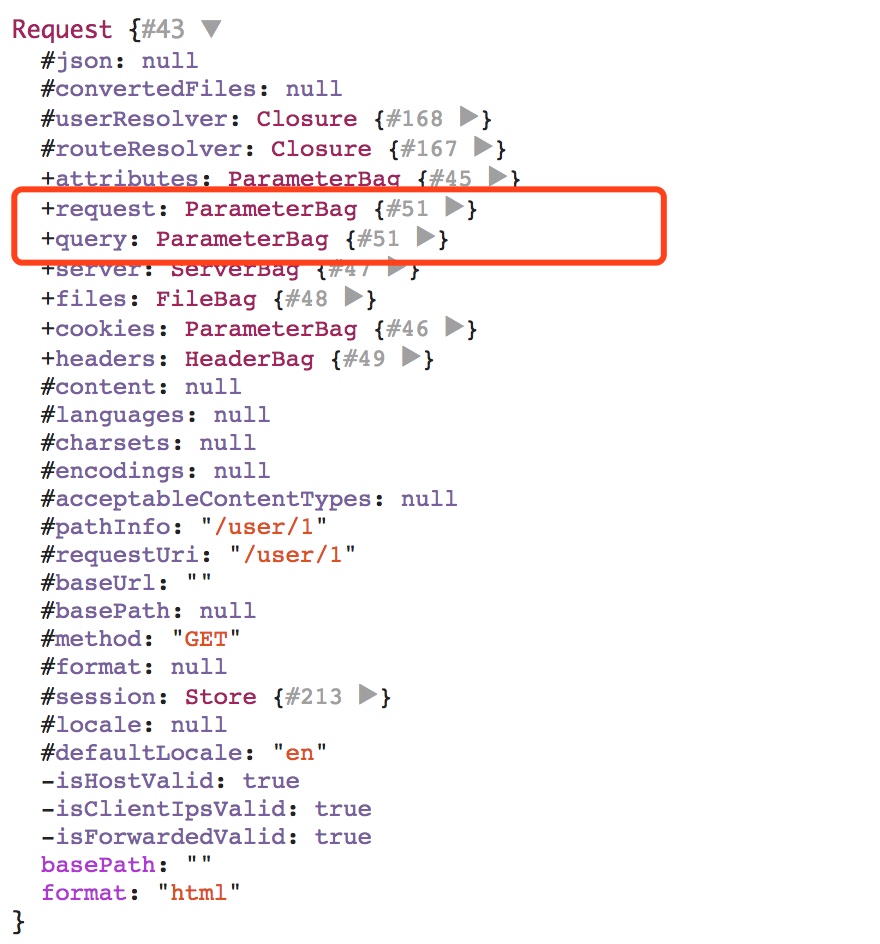
Noting the part marked with red circle, query
The way is from query
Get the parameter value in the property object, input
The method will change from query + request
Get the parameter value in the property object. There are also post
Method is used from request
The parameter value is obtained from the property object. So far, we should have some idea, query
Method is used to get GET
Request query string parameter value, input
Method is used to obtain all HTTP request parameter values, post
Method is used to get POST
Request parameter values. Interested students can go to the bottom layer to view the source code: vendor/laravel/framework/src/Illuminate/Http/Concerns/InteractsWithInput.php
。
Get input through dynamic attributes
In addition, you can also use Illuminate\Http\Request
Instance to access user input. For example, if your application form contains name
Field, you can access the submitted value like this:
$name = $request->name;
When using dynamic attributes, Larvel will first look up the value of the parameter in the request, and if it does not exist, it will also look up the routing parameter. The implementation principle of this function is naturally magic function __get
Has:
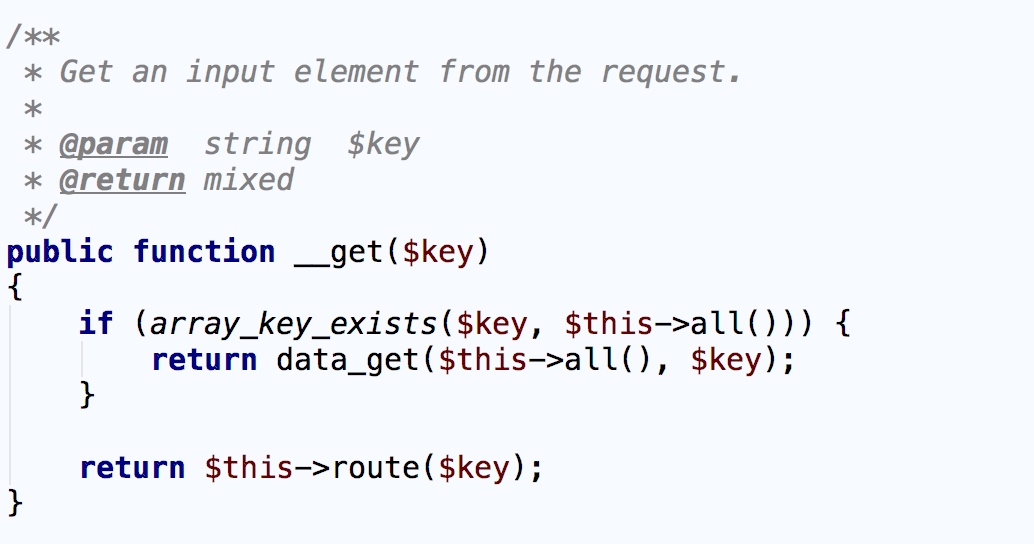
As far as I'm concerned, these grammar candies are useless and do not improve the performance. They are just easy to use, but they will also increase the cost for users to learn grammar.
Get JSON input value
When sending JSON requests to applications, as long as the Content Type request header is set to application/json
, can pass input
Method to obtain JSON data. You can also parse the array through the "." sign:
$name = $request->input('user.name');
Get part of the input data
If you need to fetch a subset of the input data, you can use only
or except
Methods, both of which accept an array or dynamic list as the only parameter, as we did in the previous article controller The syntax of the controller middleware mentioned in is similar to:
$input = $request->only(['username', 'password']); $input = $request->only('username', 'password'); $input = $request->except(['credit_card']); $input = $request->except('credit_card');
Note: only
The method returns all the parameter key value pairs you want to obtain. However, if the parameter you want to obtain does not exist, the corresponding parameter will be filtered out.
Judge whether the request parameter exists
To determine whether the parameter exists in the request, you can use has
Method, return if the parameter exists true
:
if ($request->has('name')) { // }
This method supports querying multiple parameters in the form of an array. In this case, only when the parameters all exist, will it return true
:
if ($request->has(['name', 'email'])) { // }
If you want to judge whether the parameter exists and the parameter value is not empty, you can use filled
method:
if ($request->filled('name')) { // }
Last request input
Larvel allows you to save the last input data between two requests. This feature is useful when you need to refill the form data after detecting the validation data failure. However, if you use the built-in validation function of Larvel, you do not need to use these methods manually, because some of Larvel's built-in validation settings will automatically call them. Store input to session
Illuminate\Http\Request
Instance flash
The method will store the current input in a one-time session (the so-called one-time means that the corresponding data will be destroyed from the session after the data is taken from the session), so that the data entered last time is still valid at the next request:
$request->flash();
You can also use flashOnly
and flashExcept
Methods store a subset of input data in a session. These methods are useful for storing sensitive information outside of the session. This function is applicable to scenarios where the login password is incorrectly filled in:
$request->flashOnly('username', 'email'); $request->flashExcept('password');
Store input to Session and redirect
If you often need to store the input request input at one time and return to the form filling page, you can redirect()
Called after withInput()
The method implements the following functions:
return redirect('form')->withInput(); return redirect('form')->withInput($request->except('password'));
Get the last request data
To retrieve the last requested input data from the session, you can use the old
method. old
The method can easily fetch one-time data from a session:
$username = $request->old('username');
Laravel also provides a global auxiliary function old()
If you display the last input data in the Blade template, use the auxiliary function old()
More convenient. If there is no corresponding input for the given parameter, return null
:
<input type="text" name="username" value="{{ old('username') }}">
This part of the function will be demonstrated later when we talk about view and form validation.
Cookie
Take cookies from requests
For security, all cookies created by the Larravel framework are encrypted and signed with an authentication code, which means that if the client modifies them, they need to be validated. We use Illuminate\Http\Request
Instance cookie
Method to get the value of the cookie from the request:
$value = $request->cookie('name');
Add Cookies to Response
You can use cookie
Method to add a cookie to the returned Illuminate\Http\Response
Instance, you need to pass the cookie name, value, and validity period (minutes) to this method:
Return response ('Welcome to Laravel College ') ->Cookie( 'name', 'Scholar', $minutes );
cookie
Method can accept some parameters that are used less frequently. Generally speaking, these parameters and PHP native functions setcookie
Same function and meaning:
Return response ('Welcome to Laravel College ') ->Cookie( 'name', 'Scholar', $minutes, $path, $domain, $secure, $httpOnly );
Let's briefly demonstrate the use of this function in the routes/web.php
Define two routes as follows:
Route::get('cookie/add', function () { $minutes = 24 * 60; Return response ('Welcome to Laravel College ') ->cookie ('name', 'Academic gentleman', $minutes); }); Route::get('cookie/get', function(\Illuminate\Http\Request $request) { $cookie = $request->cookie('name'); dd($cookie); });
Visit first http://blog.dev/cookie/add
Set cookies, and then go through http://blog.dev/cookie/get
Get the cookie value. If you see the output on the page Academician
, the cookie is set successfully. Of course, we can also quickly view cookie information through F12 mode of Chrome browser:
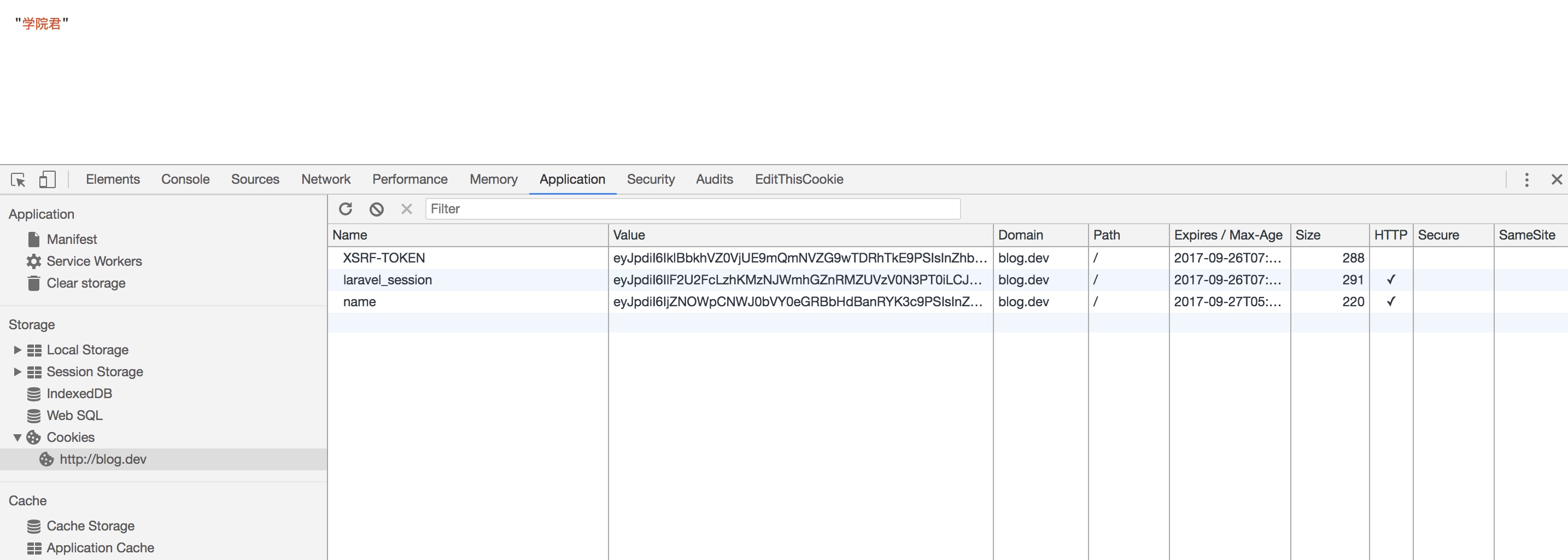
Encrypted name
The cookie we just set.
Generate Cookie Instance
If you want to generate a Symfony\Component\HttpFoundation\Cookie
Instance for subsequent addition to the response instance, you can use the global auxiliary function cookie
, this cookie will only be sent to the client when it is added to the response instance:
$cookie=cookie ('name ',' Scholar ', $minutes); Return response ('Welcome to Laravel College ') ->cookie ($cookie);
Let's rewrite the previous cookie/add
Routing implementation logic:
Route::get('cookie/add', function () { $minutes = 24 * 60; //Return response ('Welcome to Laravel College ') ->cookie ('name', 'Academic gentleman', $minutes); $cookie=cookie ('name ',' Academic X ', $minutes); Return response ('Welcome to Laravel College ') ->cookie ($cookie); });
The effect is the same as before. This visit cookie/get
Route, the page print result is Xueyuan Jun X
。
File upload
Get the uploaded file
have access to Illuminate\Http\Request
Instance provided file
Method or dynamic attribute to access the uploaded file, file
Method return Illuminate\Http\UploadedFile
An instance of a class that inherits from the SplFileInfo
Class: $file = $request->file('photo'); $file = $request->photo;
You can use hasFile
Method to determine whether the file exists in the request: if ($request->hasFile('photo')) { // }
Verify whether the file is uploaded successfully
use isValid
Method to determine whether the file is in error during uploading:
if ($request->file('photo')->isValid()){ // }
File Path&Extension
UploadedFile
Class also provides methods to access the absolute path and extension of the uploaded file. extension
The method can judge the file extension based on the file content, which may be inconsistent with the extension provided by the client:
$path = $request->photo->path(); $extension = $request->photo->extension();
Other document methods
UploadedFile
There are many other methods available on the instance. Check API document of this class Learn more.
Save the uploaded file
To save the uploaded file, you need to use a file system you have configured. The corresponding configuration is located in config/filesystems.php
: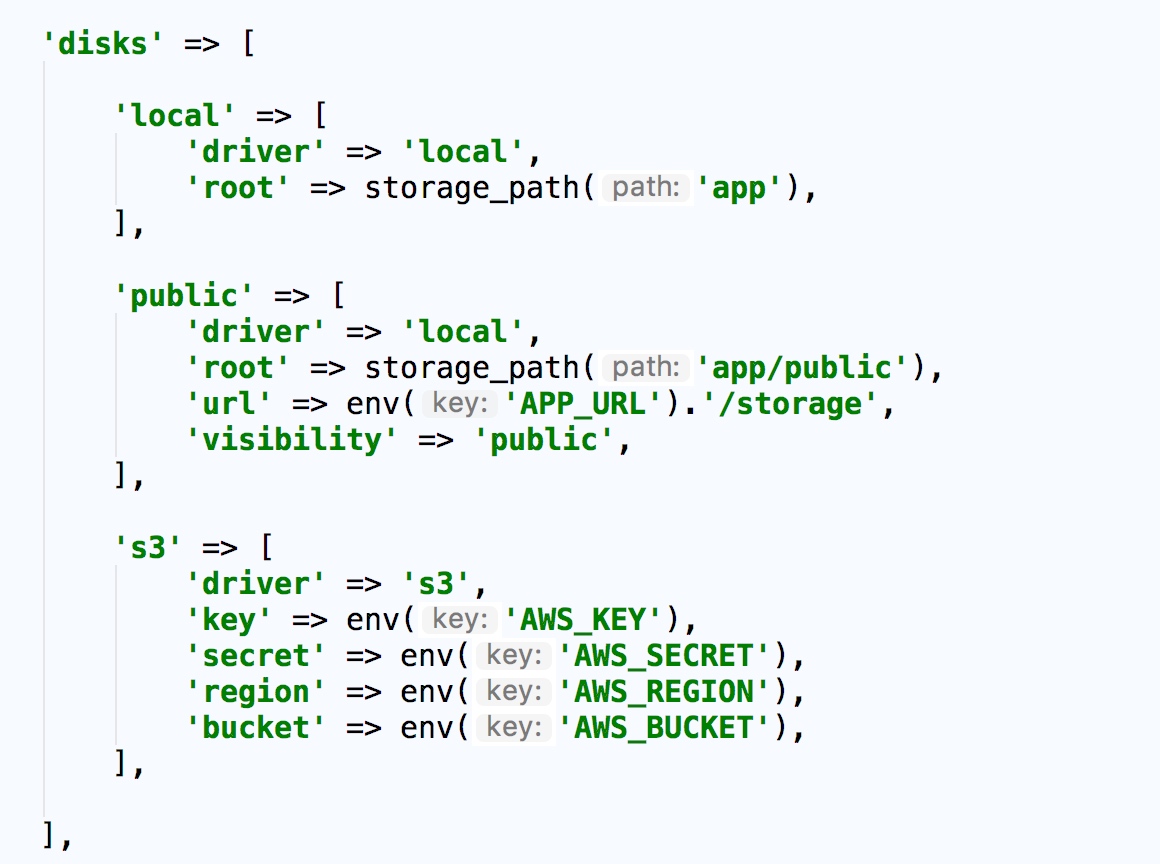
Larravel uses by default local
The configuration stores the uploaded files, that is, the local file system. The default root directory is storage/app
, public
It is also a local file system, but the files stored here can be publicly accessed, and the corresponding root directory is storage/app/public
The precondition for Web users to access the files stored in the directory is to enter the application portal public
Create a soft link under the directory storage
link to storage/app/public
。
UploadedFile
Class has a store
Method, this method will move the uploaded files to the corresponding disk path, which can be a location in the local file system or a path on cloud storage (such as Amazon S3).
store
Method receives a relative path (relative to the root directory of the file system configuration) for saving a file. The path does not need to include a file name, because the system will automatically generate a unique ID as the file name.
store
The method also receives an optional parameter -- the disk name used to store files as the second parameter (corresponding to the file system configuration disks
The default value is local
), this method will return the file path relative to the root directory:
$path = $request->photo->store('images'); $path = $request->photo->store('images', 's3');
If you don't want to automatically generate file names, you can use storeAs
Method, which receives the save path, file name, and disk name as parameters:
$path = $request->photo->storeAs('images', 'filename.jpg'); $path = $request->photo->storeAs('images', 'filename.jpg', 's3');
Let's briefly demonstrate the file upload function in routes/api.php
The following file upload routes are defined in:
Route::post('file/upload', function(\Illuminate\Http\Request $request) { if ($request->hasFile('photo') && $request->file('photo')->isValid()) { $photo = $request->file('photo'); $extension = $photo->extension(); //$store_result = $photo->store('photo'); $store_result = $photo->storeAs('photo', 'test.jpg'); $output = [ 'extension' => $extension, 'store_result' => $store_result ]; print_r($output); exit(); } Exit ('No uploaded file was obtained or an error occurred during the upload process'); });
We still use the Advanced REST Client tool to demonstrate POST form submission:
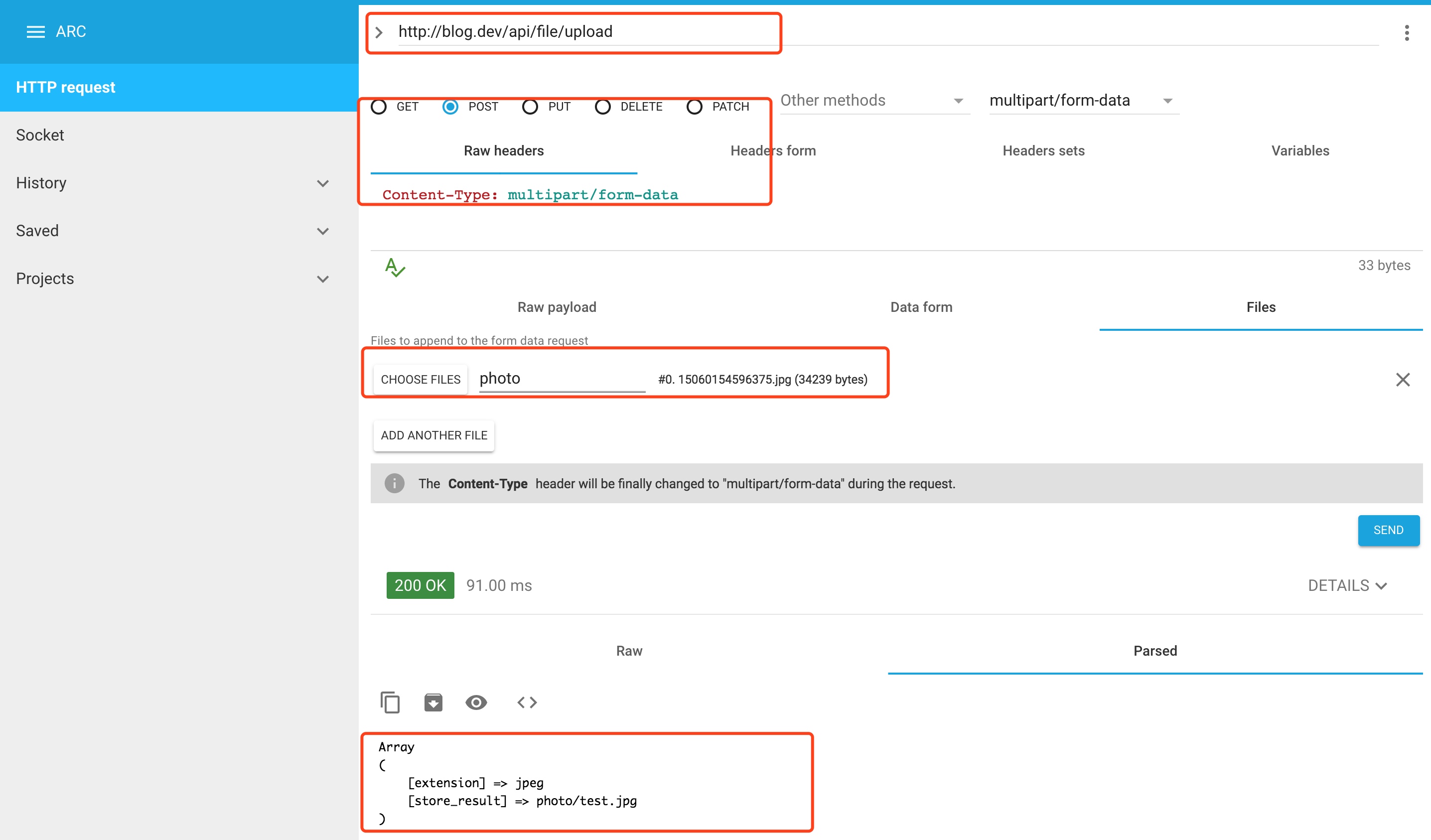
The places marked with red circles are the inputs and outputs that need to be focused on. I tested them separately store
Methods and storeAs
Method. You can go to storage/app
View under directory:
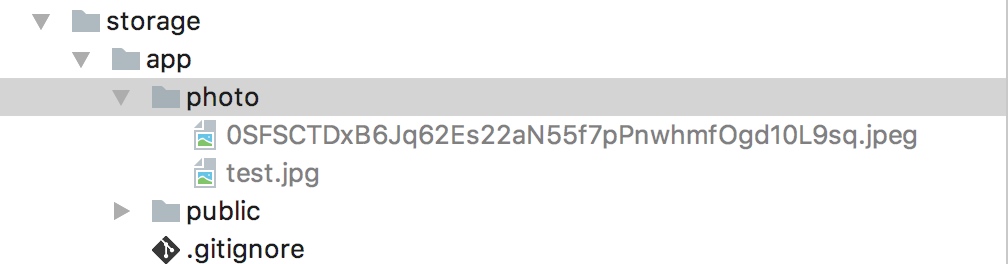
Other storage media are used in the same way, just under modification store
and storeAs
Corresponding parameters. If you encounter any problems in the use process, please give feedback in the comment area.
Configure trust agent
If your application runs behind a load balancer that will interrupt the TLS/SSL certificate, you will notice that sometimes the application will not generate HTTPS links. Usually, this is because the application is forwarding traffic from the load balancer from port 80, so you do not know that it should generate secure encrypted links. To solve this problem, you can use the new App\Http\Middleware\TrustProxies
Middleware, which allows you to quickly customize the load balancer or agent that needs to be trusted by the application. The trusted proxy is located in the $proxies
Attribute list. In addition to configuring the trust agent, you can also configure the message headers sent by the agent with the request source information:

Trust all agents
If you are using load balancing provided by Amazon AWS or other cloud services and do not know the real IP address of the equalizer, you can use **
Wildcards trust all agents:
/** * The trusted proxies for this application. * * @var array */ protected $proxies = '**';