Use the middleware VerifyCsrfToken to avoid CSRF attacks

Introduction&Function Demonstration
Cross site request forgery (CSRF) is a malicious vulnerability that attacks credit websites by masquerading requests from authorized users. Larvel makes it easy to avoid cross site request forgery attacks on applications through its own CSRF protection middleware: Larvel will automatically generate a CSRF "token" for each effective user session managed by the application, and then store the token in the session. The token is used to verify whether the authorized user and the initiator are the same person.
Whenever you define an HTML form in the Larravel application, you need to introduce a CSRF token field in the form, so that the CSRF protection middleware can verify the request. To generate a hidden input field containing a CSRF token, you can use an auxiliary function csrf_field
:
<form method="POST" action="/profile"> {{ csrf_field() }} ... </form>
Middleware group web
Middleware in VerifyCsrfToken
It will automatically verify for us whether the token value entered in the request is consistent with the token stored in the session. If the field is not passed or the field value passed is inconsistent with the value stored in the session, an exception will be thrown.
To demonstrate this feature, we have routes/web.php
Define a set of test routes in:
Route::get('form_without_csrf_token', function (){ Return '<form method="POST" action="/hello_from_form"><button type="submit">Submit</button></form>'; }); Route::get('form_with_csrf_token', function () { Return '<form method="POST" action="/hello_from_form">'. csrf_field(). '<button type="submit">Submit</button></form>'; }); Route::post('hello_from_form', function (){ return 'hello laravel!'; });
We access http://blog.dev/form_without_csrf_token
And click the submit button on the page, the page reports an error and throws MethodNotAllowedHttpException
Exception, which usually means that the CSRF token field is not passed or the passed token field is incorrect:
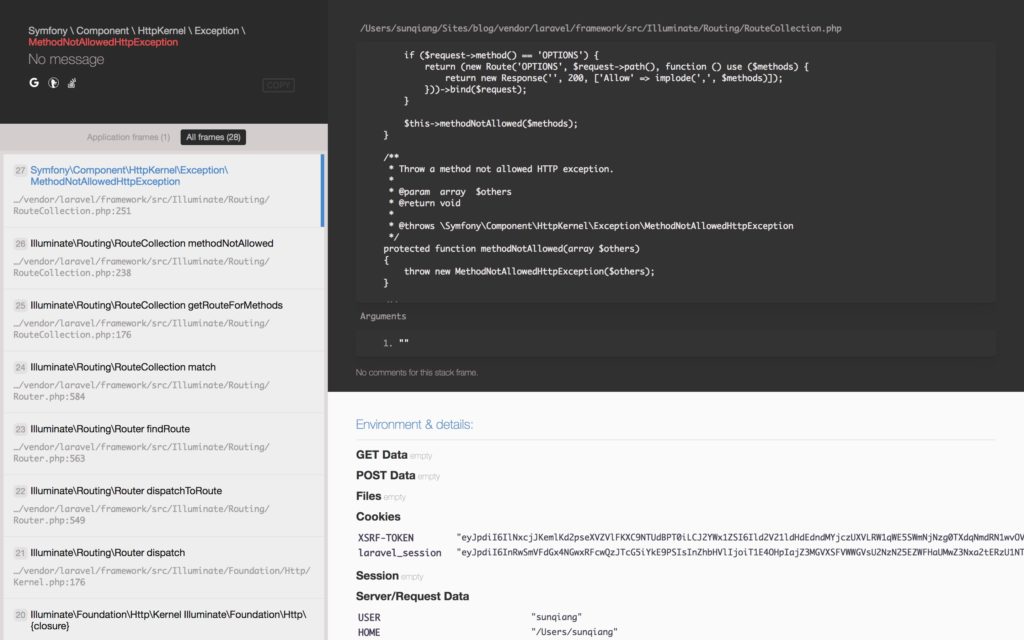
And when we visit http://blog.dev/form_csrf_token
When you click the Submit button on the page, the page displays normally.
Note: CSRF middleware only works for routes/web.php
Because the routes under this file are assigned web
Middleware group, while VerifyCsrfToken
be located web
Middleware group.
Exclude the specified URL from CSRF security verification
Sometimes we need to exclude some URLs from the CSRF protection middleware. For example, if you use a third-party payment system (such as Alipay or WeChat payment) to process payment and use the callback function provided by them, then you need to exclude the callback processor route from Larvel's CSRF protection middleware, Because the third-party payment system does not know what token value to send to our defined route. Usually we need to put this type of route to a file routes/web.php
In addition, such as routes/api.php
。 However, if it is necessary to add routes/web.php
If you are in, you can also VerifyCsrfToken
Add the URL to be excluded to $except
Attribute array:
<? php namespace App\Http\Middleware; use Illuminate\Foundation\Http\Middleware\VerifyCsrfToken as Middleware; class VerifyCsrfToken extends Middleware { /** *URLs excluded from CSRF validation * * @var array */ protected $except = [ 'alipay/*', ]; }
X-CSRF-Token
In addition to verifying the CSRF token as a POST parameter, you can also set the X-CSRF-Token
The request header is used for verification, VerifyCsrfToken
Middleware will check X-CSRF-TOKEN
Request header. The implementation method is as follows: first, create a meta tag and save the token to the meta tag: <meta name="csrf-token" content="{{ csrf_token() }}">
Then add the token to all request headers in the js library (such as jQuery), which provides a simple and convenient way for AJAX based requests to avoid CSRF attacks:
$.ajaxSetup({ headers: { 'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content') } });
CSRF token and JavaScript
When building JavaScript driven applications, it is convenient to add a CSRF token field to each exit request of the JavaScript HTTP library. By default, resources/assets/js/bootstrap.js
The file has been registered through the Axios HTTP library csrf-token
The value of this meta tag is used as the token field value. If you do not use this library, you need to manually configure it to achieve similar functions.
X-XSRF-Token
Larave will also save the CSRF token to a file named XSRF-TOKEN
In the cookie, you can use the cookie value to set X-XSRF-TOKEN
Request header. Some JavaScript frameworks, such as Angular and Axios, will automatically set this value for you. Basically, you don't need to set this value manually.
last, VerifyCsrfToken
The underlying implementation source code of the middleware framework is located in vendor/laravel/framework/src/Illuminate/Foundation/Http/Middleware/VerifyCsrfToken.php
, interested students can go to have a look.