Entrance to the Larravel application: Routing Model Binding of Routing Series
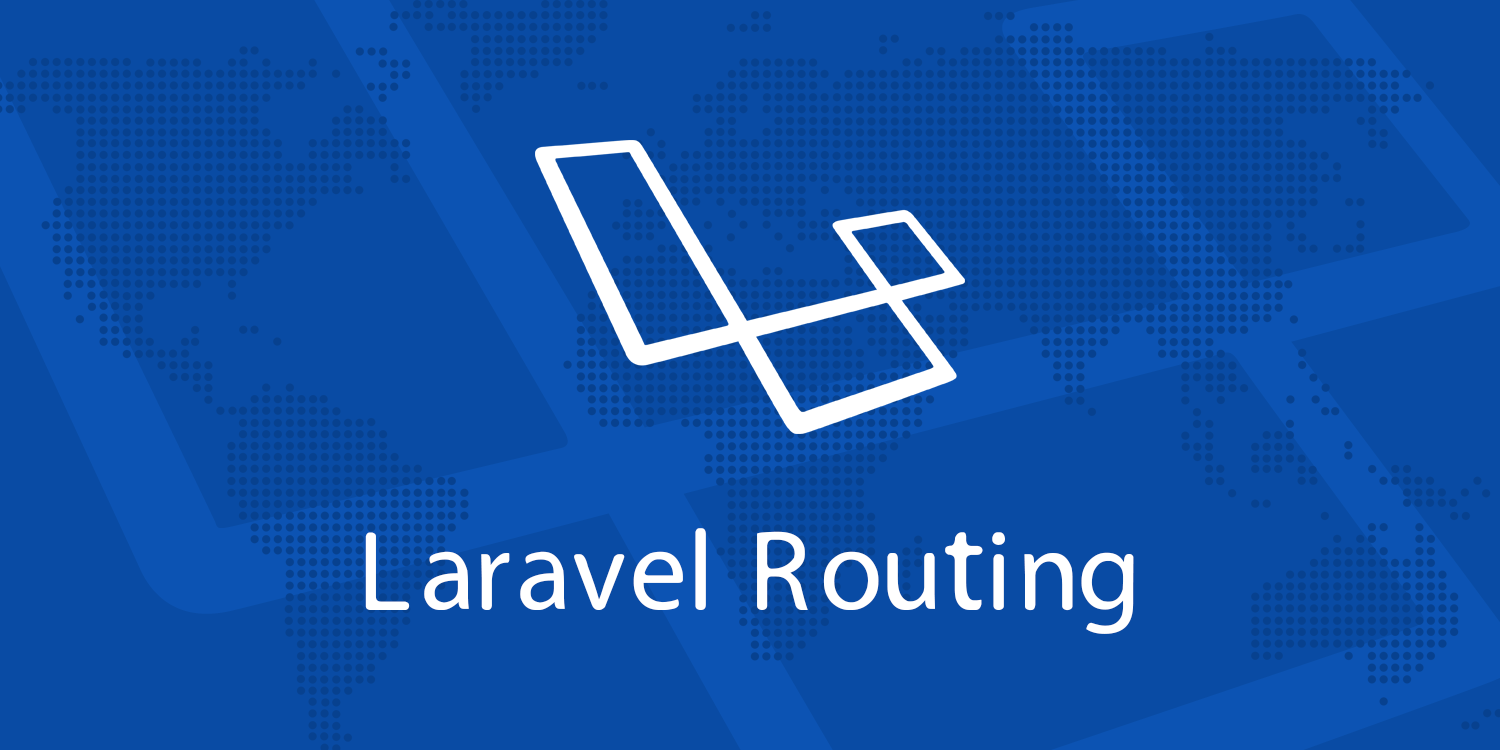
Routing model binding
When injecting a model ID to a route or controller action, you usually need to query the database to obtain the corresponding model data. The Larravel routing model binding makes it easy to inject model instances to routes. For example, you can match the entire User
Class instances are injected into the route, not just the user ID.
Implicit binding
Laravel will automatically parse the Eloquent model type declaration defined in the route or controller action (variable name matches the route fragment), for example (we define this route in routes/api.php
In the document):
Route::get('users/{user}', function (App\User $user) { dd($user); });
In this example, because the type declares the Eloquent model App\User
, corresponding variable name $user
Will match the {user}
In this way, Larvel will automatically inject the user model instance corresponding to the ID passed in the request URI.
Before demonstrating this function, we need to create a data table. Since I developed in the Valet development environment, we need to create a database ourselves. We name the database valet
, the local database user name is root
, password is empty, modify accordingly .env
The file configuration is as follows:
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=valet DB_USERNAME=root DB_PASSWORD=
The specific configuration values are subject to your own development environment settings. We will create it based on the powerful database migration function of Laravel users
Table. The database migration will be discussed in detail later in the database section. Here we use the following command to generate users
Table:
php artisan migrate
Entering the database, you can see that the table has been generated:

At this time, users
The data table does not have any records. If the corresponding model instance cannot be found in the database, an HTTP 404 response will be automatically generated, prompting that the page does not exist, so we need to insert a record in this table. Here we can quickly complete the data filling function based on the powerful database filler of Laravel. First, we can generate the following command users
Corresponding data table filler:
php artisan make:seeder UsersTableSeeder
This command will generate a UsersTableSeeder
File, edit the file as follows:

Then edit the DatabaseSeeder.php
The file is as follows (cancel the comment before calling the data table filler):

Final execution php artisan db:seed
The corresponding data can be inserted into users
Table, so we can access it again in the browser http://blog.dev/api/users/1
Will be displayed when User
Model data:

Next, you can directly take $user
The model does what you want to do instead of querying the database itself, thus improving the efficiency of development.
Custom Key Name
If you want to use other fields of the data table instead of id
Field, which can override the getRouteKeyName
Method to User
For example, you can add this method to the model class:
/** * Get the route key for the model. * * @return string */ public function getRouteKeyName() { return 'name'; }
So we can get through http://blog.dev/api/users/jroJoGP71W
Access the same model instance. It should be noted here that if the field is not a unique key, the first record in the result set will be returned. The corresponding underlying implementation is here:

Explicit binding
If there is implicit binding, there is explicit binding. To register explicit binding, you can use the router's model
Method to specify a binding class for the given parameter. You need to RouteServiceProvider
Class boot
Define explicit model binding in the method:
public function boot() { parent::boot(); Route::model('user_model', \App\User::class); }
Next, in routes/api.php
Define a include in {user}
Route of parameters:
$router->get('profile/{user_model}', function(App\User $user) { dd($user); });
Because we have already bound {user_model}
Parameters to App\User
Model, User
The instance will be injected into the route. Therefore, if the request URL is http://blog.dev/api/profile/1
, a user ID of one
Of User
example.
If the matched model instance does not exist in the database, an HTTP 404 response will be automatically generated and returned.
Custom parsing logic
If you want to use custom parsing logic, you can RouteServiceProvider
Class boot
Method Route::bind
Method, passing to bind
The closure of the method will get the value in the URI request parameter and return the class instance you want to inject in the route:
public function boot() { parent::boot(); Route::bind('user', function($value) { return \App\User::where('name', $value)->first(); }); }
With these methods, you can basically meet your various requirements for routing model binding.
Access the current route
You can use Route
On the facade current
、 currentRouteName
and currentRouteAction
Method to access the routing information for processing the current input request:
//Get the current routing instance $route = Route::current(); //Get the current route name $name = Route::currentRouteName(); //Get the current route action attribute $action = Route::currentRouteAction();
Refer to the API documentation Routing front bottom class as well as Route instance More available methods for.
Route cache
Note: Route caching does not apply to closure based routes. To use route caching, you must convert closure routes to controller routes.
If your application is completely based on the controller route, you can use Laravel's route cache. Using the route cache will greatly reduce the time cost of registering all application routes. In some cases, the speed of route registration can even be increased by 100 times! To generate a route cache, simply execute the Artisan command route:cache
:
php artisan route:cache
After running, each request will read the route from the cache, so if you add a new route, you need to regenerate the route cache. Therefore, it is only necessary to run in the project deployment phase route:cache
Command, the local development environment is completely unnecessary.
To remove the cache route file, use route:clear
Command:
php artisan route:clear
We will discuss controller routing in detail in the controller section.