Entrance to the Larravel application: Basic Introduction to Routing Series
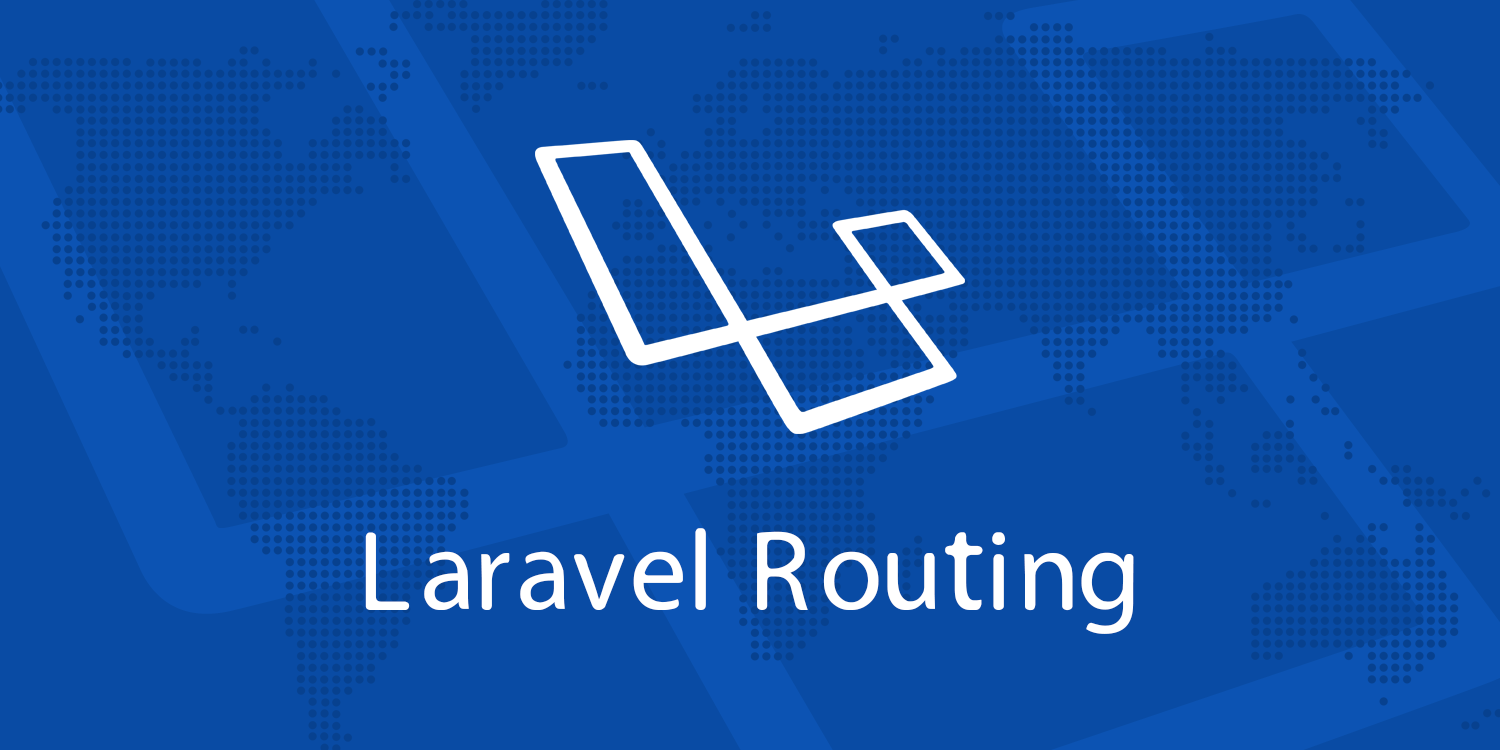
Basic route
The most basic Laravel route only receives one URI and one closure, and provides a very simple and elegant route definition method based on this: Route::get('hello', function () { return 'Hello, Welcome to LaravelAcademy.org'; });
We take Installation Configuration New in document blog
For example, in the routes/web.php
Define the route in: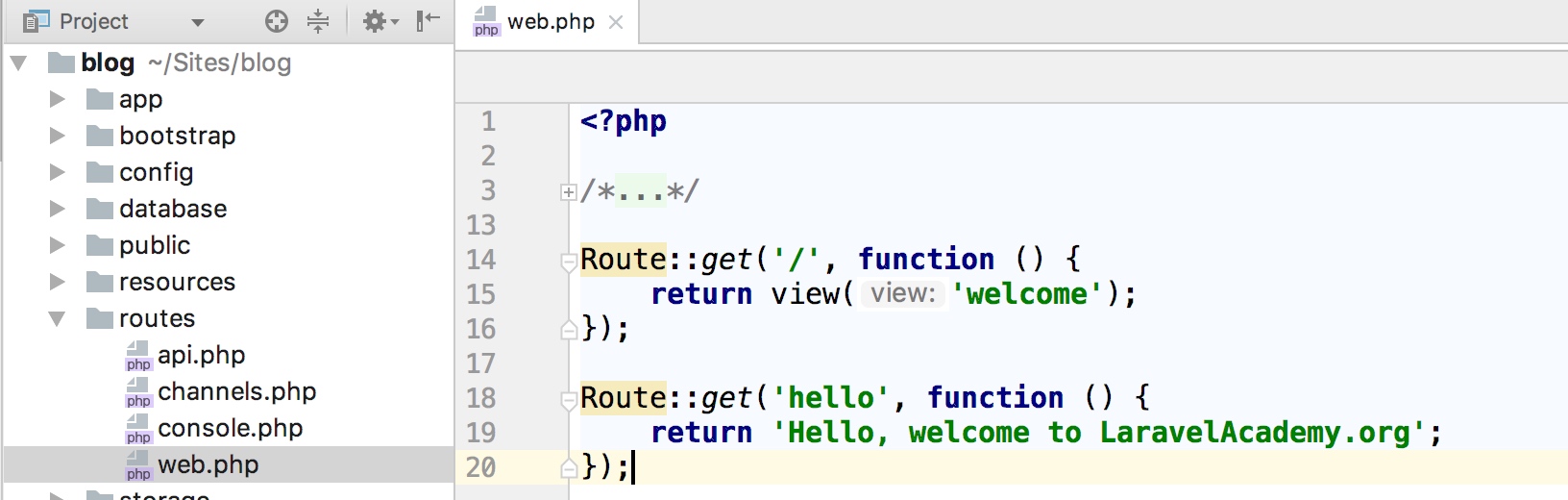
In the browser through http://blog.dev/hello
(I use Valet as the development environment, so the corresponding domain name is blog.dev
, the actual domain name is subject to your own configuration), you can access the route we just defined, and the page output is as follows:
Hello, welcome to LaravelAcademy.org
Default routing file
All Laravel routes are defined in the routes
In the routing files under the directory, these files are automatically loaded through the framework, and the corresponding logic is located in app/Providers/RouteServiceProvider
Class (we will discuss this part of logic in detail when we talk about the Laravel startup process later). routes/web.php
The file defines the routes of the Web interface, which are assigned to web
Middleware group, so that session, csrf protection and other functions can be used. routes/api.php
Routes in are stateless because they are assigned to api
Middleware group. For most applications routes/web.php
The file starts to define the route. Defined in routes/web.php
Routes in can be accessed by entering the corresponding URL in the browser address bar. For example, you can http://blog.dev/user
Access the following routes:
Route::get('/user', ' UsersController@index ');
As mentioned earlier, the definition is routes/api.php
Routes in files pass through app/Providers/RouteServiceProvider
The processing of is nested in a routing middleware group. In this routing middleware group, all routes will be automatically added /api
Prefix, so you don't need to manually add each route to the route file. You can edit RouteServiceProvider
Class to modify the routing prefix and other routing middleware group options:
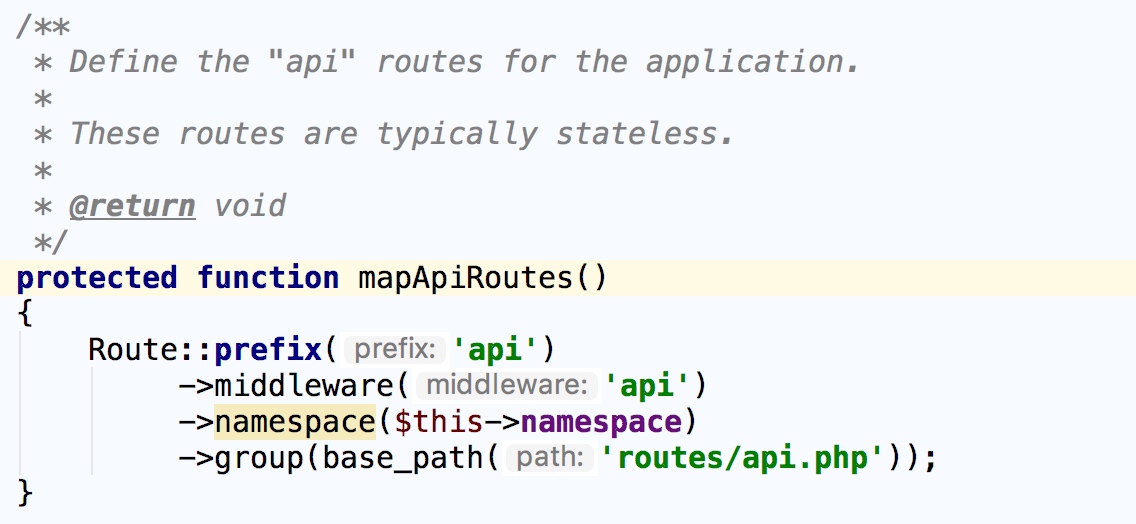
Supported request methods
We can register a route to respond to any HTTP request action: Route::get($uri, $callback); Route::post($uri, $callback); Route::put($uri, $callback); Route::patch($uri, $callback); Route::delete($uri, $callback); Route::options($uri, $callback);
Sometimes you also need to register a route to respond to multiple HTTP request actions - this can be done through match
Method. Alternatively, you can use any
Method registers a route to respond to all HTTP request actions: Route::match(['get', 'post'], 'foo', function () { return 'This is a request from get or post'; }); Route::any('bar', function () { return 'This is a request from any HTTP verb'; });
When testing GET requests, you can directly enter the request address in the browser. Testing POST requests can be done through client tools, such as Advanced REST Client, which can be downloaded from the Chrome App Store. In addition, if the above route is defined in the routes/web.php
Before testing the POST request, you need to cancel the CSRF protection check for the corresponding route, or it will return four hundred and nineteen
The status code causes the request to fail. The cancellation method is app/Http/Middleware/VerifyCsrfToken
Set the exclusion check route in:
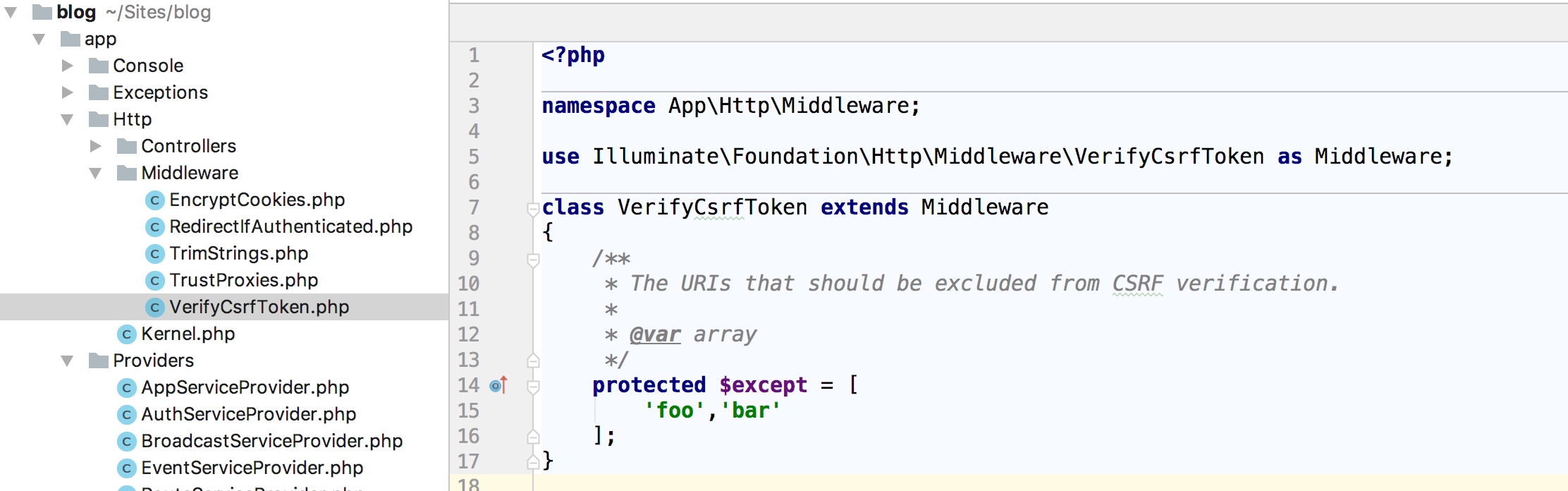
Let's test the POST request:
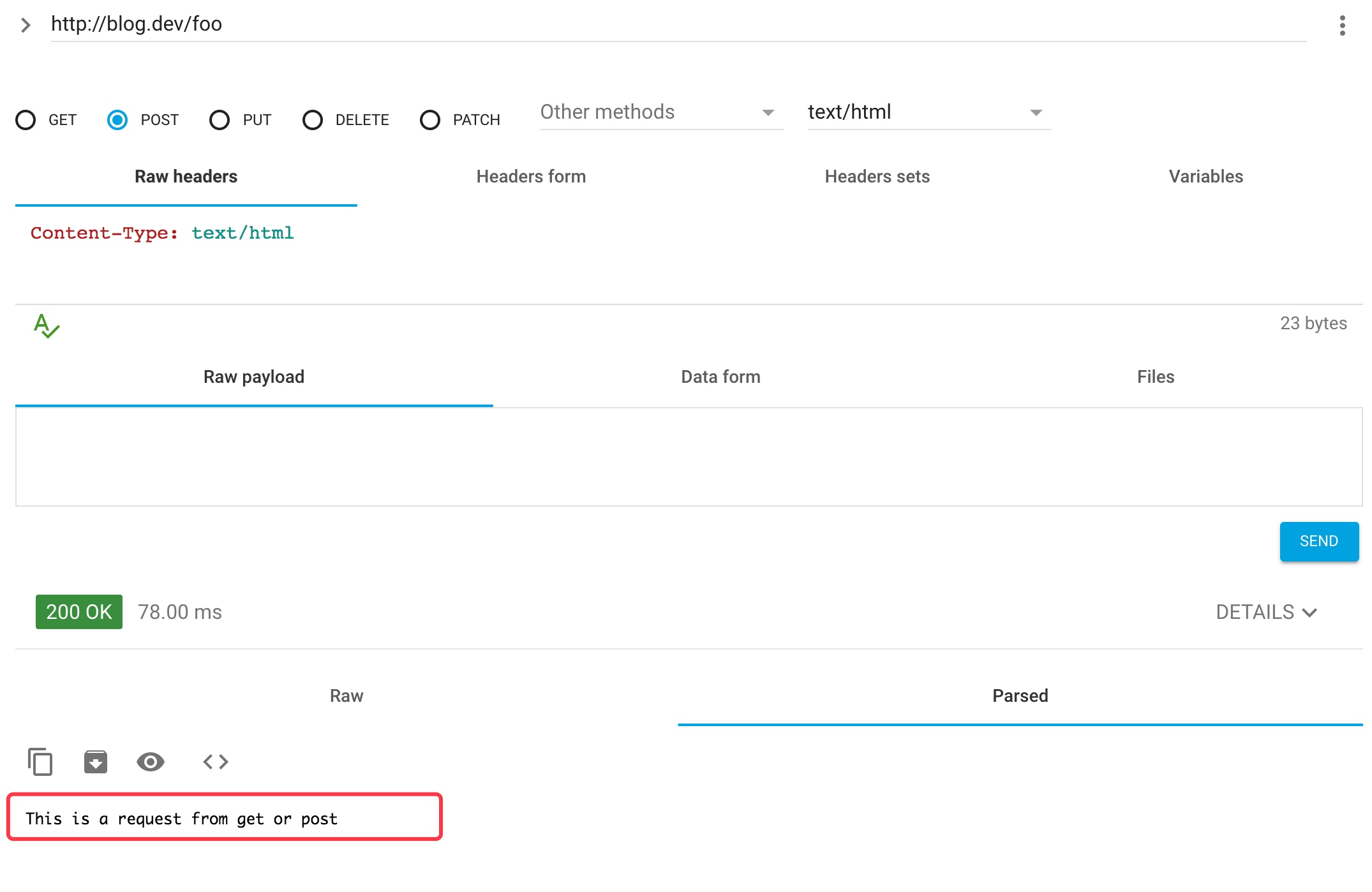
If the route is defined in routes/api.php
If so, there is no need to pay attention to CSRF protection. For example, we routes/api.php
definition bar
Routing, and in VerifyCsrfToken
Of $except
Remove from property array bar
, and then we test http://blog.dev/api/bar
POST requests for:
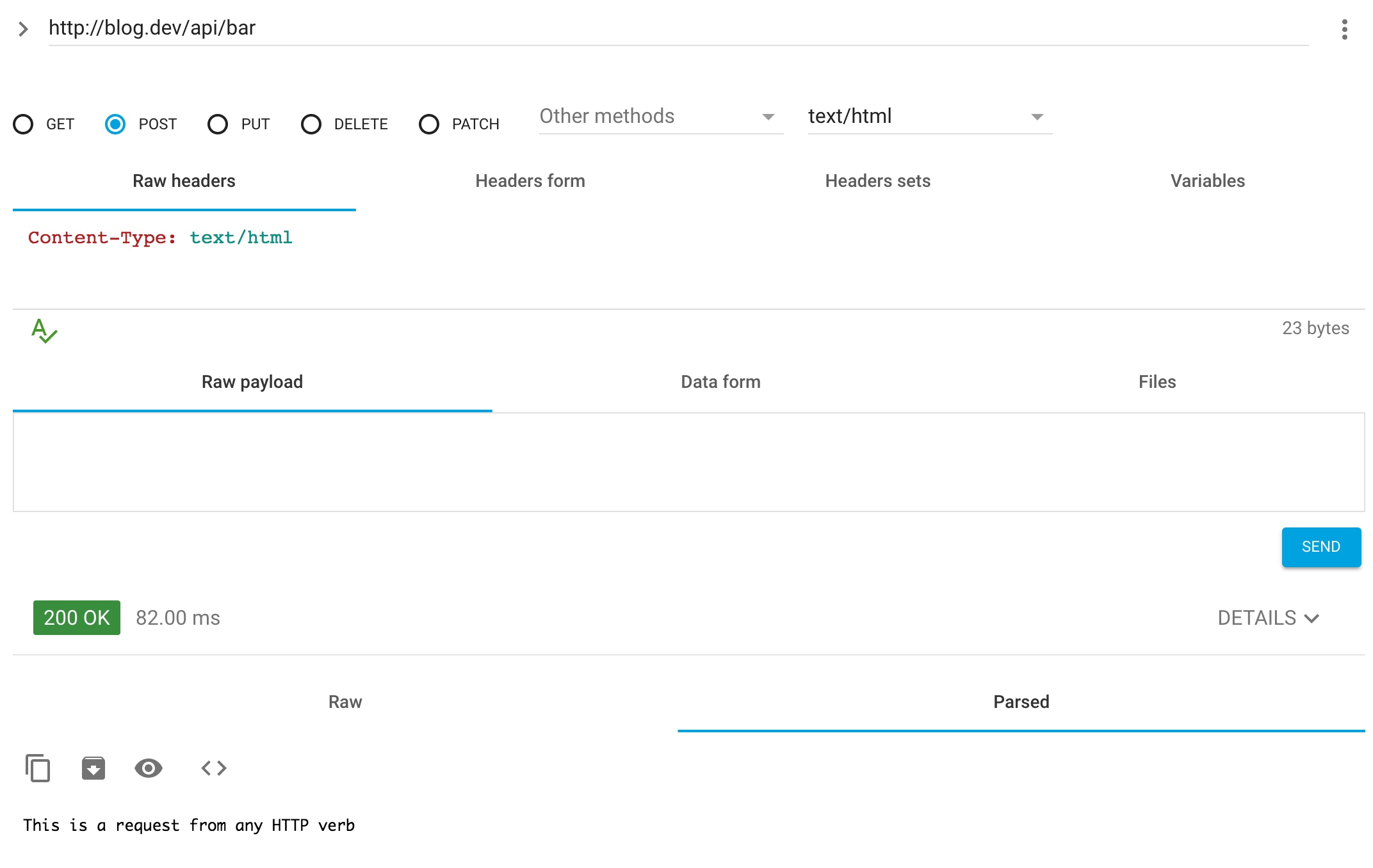
As we predicted, there is no problem at all. The reason behind this is that web
All routes defined in the route file are located in web
Middleware group, which enables CSRF protection check by default, and api
Routing files are located in api
Middleware group. The routing under this middleware group is mainly used for third-party API requests. CSRF check cannot be performed, so no processing is required.
CSRF protection
stay routes/web.php
All request modes in the routing file are PUT
、 POST
or DELETE
The HTML form corresponding to the route of must contain a CSRF token field, otherwise, the request will be rejected. For more details about CSRF, please refer to CSRF Document : <form method="POST" action="/profile"> {{ csrf_field() }} ... </form>
Or above foo
For example, if we are not in VerifyCsrfToken
The middleware excludes its inspection (in fact, such operations are also unsafe), so it needs to be carried in the form submission csrf_token
Field:

So when we visit http://blog.dev/form
Then click the submit button on the page, and the page will jump to http://blog.dev/foo
The following contents are displayed:
This is a request from get or post
Form method forgery
HTML forms are not supported PUT
、 PATCH
perhaps DELETE
Request method, so call the PUT
、 PATCH
or DELETE
When routing, you need to add a hidden _method
Field whose value is used as the HTTP request method of the form: <form action="/foo/bar" method="POST"> <input type="hidden" name="_method" value="PUT"> <input type="hidden" name="_token" value="{{ csrf_token() }}"> </form>
You can also use auxiliary functions directly method_field
To realize this function:
{{ method_field('PUT') }}
Route Redirection
If you need to define a route to redirect to other URIs, you can use Route::redirect
This method is so convenient that you don't need to define additional routes or controllers to execute simple redirection logic: Route :: redirect ( '/here' , '/there' , three hundred and one );
among here
Indicates the original route, there
Indicates the route after redirection, three hundred and one
It is an HTTP status code used to identify redirection. Routing View
If your route needs to return a view, you can use Route::view
Methods, and redirect
The method is similar. This method is also very convenient, so you don't need to define an additional route or controller. view
The method receives a URI as the first parameter and a view name as the second parameter. In addition, you can also provide an array data to pass to the view method as an optional third parameter, which can be used for data rendering in the view: Route :: view ( '/welcome' , 'welcome' ); Route :: view ( '/welcome' , 'welcome' , [ 'name' => 'Academician' ]);
We define a route view in routes/web.php as follows:

To ensure that it can be shared welcome.blade.php
For this view file, we also provide /
The route has been adjusted. Next, we need to modify resources/views/welcome.blade.php
Code to support website
Data variable:


We will write the original dead Laravel
The text is adjusted to support variable input, so that we can use the http://blog.dev/view
Access the routing view:
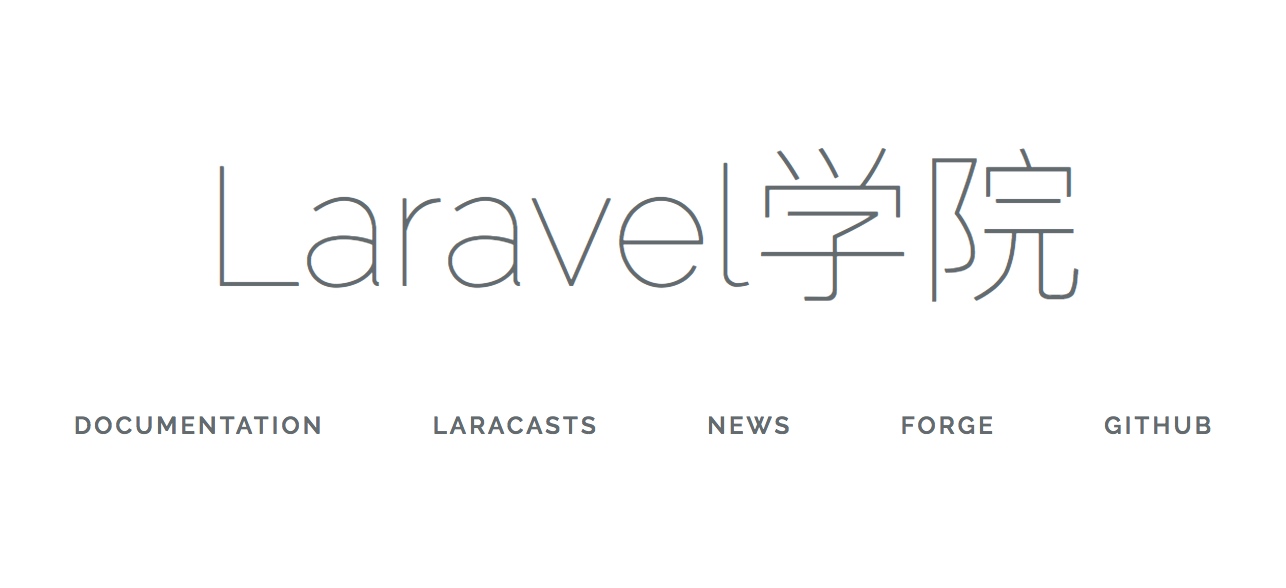
