Implementation of binary tree storage mode, traversal deletion and other functions
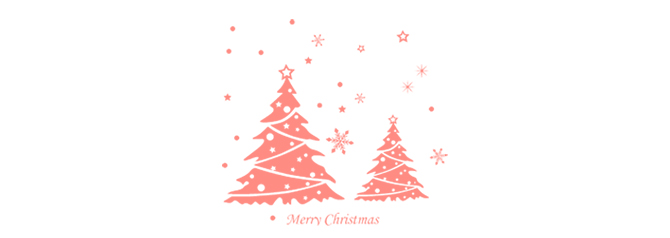
1、 Continuous stored binary tree
1. Declare a complete binary tree class
class BTreeArray<T> { private T[] bTree; private int count; public BTreeArray(int _number) { count = 0; bTree = new T[_number]; } public bool Add(T _item) { //Storage full if (count >= bTree. Length) { ShowInfo ("storage space is full"); return false; } //Store data bTree[count++] = _item; return true; } private void ShowInfo(string _str) { StringBuilder sb = new StringBuilder(); sb.Append("BTree:"); sb.Append(_str); Console.WriteLine(sb.ToString()); } }
2. Complete binary tree traversal
A Preorder traversal (DLR)
private void BTreeDLR(int _index) { //Node number is 1 greater than array index int index = _index - 1; //When the index is greater than the total number, the node does not exist if (index >= count) { return; } //Access yourself first Console.WriteLine(bTree[index]); //Access the left child node BTreeDLR(_index * 2); //Access the right child node BTreeDLR(_index * 2 + 1); } public void BTreeDLR() { BTreeDLR(1); }
int[] data = {10,78,9,56,12,11}; BTreeArray<int> bTree = new BTreeArray<int>(data. Length); for (int i = 0; i < data.Length; i++) { bTree.Add(data[i]); } bTree.BTreeDLR(); Console.ReadKey();
B Middle order traversal (LDR)
private void BTreeLDR(int _index) { //Node number is 1 greater than array index int index = _index - 1; //When the index is greater than the total number, it indicates that the end of the road has been reached if (index >= count) { return; } //Access the left child node BTreeLDR(_index * 2); //Access self Console.Write(bTree[index] + " "); //Access the right child node BTreeLDR(_index * 2 + 1); } public void BTreeLDR() { BTreeLDR(1); }
bTree.BTreeLDR();
C Post order traversal (LRD)
private void BTreeLRD(int _index) { int index = _index - 1; if (index >= count) { return; } BTreeLRD(_index * 2); BTreeLRD(_index * 2 + 1); Console.Write(bTree[index] + " "); } public void BTreeLRD() { BTreeLRD(1); }
bTree.LRD();
2、 Binary tree stored in linked list
1. Declare sorting binary tree
class BTreeLink { private BTreeLinkNode<int> headNode; public BTreeLink(BTreeLinkNode<int> _root) { headNode = _root; } } class BTreeLinkNode<T> where T:struct { public BTreeLinkNode<T> Parent { get; set; } public BTreeLinkNode<T> LChild { get; set; } public BTreeLinkNode<T> RChild { get; set; } public T Data { get; set; } public BTreeLinkNode(T _data) { Data = _data; } }
2. Sort Binary Tree Add Node
public bool Add(int _data) { Console. WriteLine ("to add"+_data); BTreeLinkNode<int> node = new BTreeLinkNode<int>(_data); if (headNode == null) { headNode = node; return true; } if (node == null) { return false; } BTreeLinkNode<int> temp = headNode; while (true) { if (node. Data <= temp.Data) { Console. WriteLine ("Check, the added value is less than"+temp. Data); //Less than, placed at the left node if (temp.LChild == null) { Console. WriteLine ("The current node has no left child node"); //Left node is empty temp.LChild = node; node.Parent = temp; break; }else { temp = temp.LChild; } } else if (node. Data > temp.Data) { Console. WriteLine ("Check, the added value is greater than"+temp. Data); if (temp.RChild == null) { Console. WriteLine ("The current node has no right child node"); //The right node is empty temp.RChild = node; node.Parent = temp; break; }else { temp = temp.RChild; } } } return true; }
3. Sort the binary tree to find the node
public BTreeLinkNode<int> Find(int _data) { BTreeLinkNode<int> temp = headNode; while (true) { if (temp == null) { //Not found return null; } if (temp.Data == _data) { //Find return temp; } if (_data > temp.Data) { //Find Right temp = temp.RChild; }else { //Find Left temp = temp.LChild; } } }
public BTreeLinkNode<int> Find(int _data, BTreeLinkNode<int> _node) { if (_node == null) { //Jump out Console. WriteLine ("BTree: no node with the value of"+_data+"was found"); return null; } if (_data == _node. Data) { return _node; } else if (_data < _node. Data) { _node = _node. LChild; }else { _node = _node. RChild; } //Recursion return Find(_data, _node); }
BTreeLinkNode testNode = bTreeLink.Find(19); BTreeLinkNode testNode1 = bTreeLink.Find(15, root); if (testNode != null && testNode1 != null) { Console. WriteLine ("Found"+testNode Data); Console. WriteLine ("Found"+testNode1. Data); }
4. Sort Binary Tree Delete Node
public bool Del(int _data) { BTreeLinkNode<int> temp = Find(_data); if (temp == null) { Console. WriteLine ("BTree:: Del: deletion failed, there is no node with the value of"+_data+"); return false; } return Del(temp); } public bool Del(BTreeLinkNode<int> _node) { BTreeLinkNode<int> temp; if (_node. LChild == null && _node.RChild == null) { //Leaf node, delete directly if (_node. Data > _node.Parent.Data) { _node. Parent.RChild = null; } else { _node. Parent.LChild = null; } _node. Parent = null; return true; } else if (_node. LChild != null) { temp = _node.LChild; while (temp.RChild != null) { temp = temp.RChild; } //Find the maximum value on the left and place it at this position _node. Data = temp.Data; Del(temp); } else if (_node. RChild != null) { temp = _node.RChild; while (temp.LChild != null) { temp = temp.LChild; } //Find the minimum value on the right and place it here _node. Data = temp.Data; Del(temp); } return true; }
bTreeLink.Del(20); if (bTreeLink. Find(20) == null) { Console. WriteLine ("20 GG"); }
5. Modify the sorting binary tree node
6. Sort binary tree traversal
private void DLR(BTreeLinkNode<int> _node) { if (_node == null) { return; } Console.Write(_node. Data +" "); DLR(_node. LChild); DLR(_node. RChild); } public void DLR() { DLR(headNode); }
bTreeLink.DLR();
summary
References
Comment
Comment area
0F Got it, next one (funny) 0F Okay, pretend you understand~ +1 Pretend to understand 23333
0F Another algorithm boss 0F Does the boss engage in acm 0F Continuous storage is mainly due to the unreasonable use of space. If the table is full, you have to find a way to apply for new space, which is often twice the current size. After the application is completed, the data must be migrated again, which is a lot of trouble. However, the advantage is fast and easy to install cache. However, it is impossible to make the linked list fast, and it is difficult to install the cache. It is just like accessing the memory frequently. But you can combine the two. 0F Rabbit GG (funny 0F Too advanced to understand. Let's say Happy New Year's Day! 0F I don't know anything after reading 2333