The Difference between Array Name and Pointer in C Language and a Simple Bubble Sort
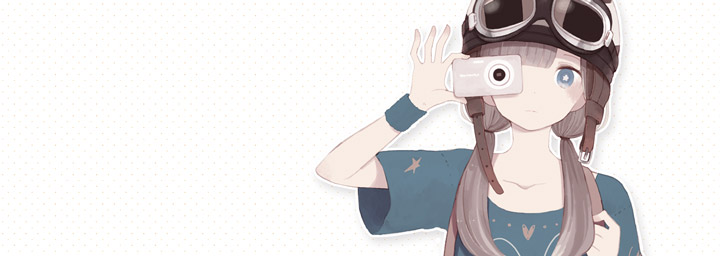
cat
Problem recurrence
int * maoPao(int *a); int main() { int a[5] = {21,3,12,9,5}; int *p = maoPao(a); printf("%d %d %d %d %d",p[0],p[1],p[2],p[3],p[4]); } int * maoPao(int *p) { int len = sizeof(p)/sizeof(p[0]); Printf ("Number of array elements% d r n", len); //Bubble sort for(int i = 0; i < len - 1; i++) { int flag = 1; for(int j = 0 ; j < len - 1 -i;j++) { if(p[j] > p[j+1]) { int t = p[j]; p[j] = p[j+1]; p[j+1] = t; flag = 0; } } if(flag) { break; } } //Void can also be used, because the operations are all pointer related, and the value of array a has changed. return p; }
Number of array elements 2 3 21 12 9 5
solve the problem
int * maoPao(int *p,int arrLen) { int len = arrLen; Printf ("Number of array elements% d r n", len); //Bubble sort for(int i = 0; i < len - 1; i++) { int flag = 1; for(int j = 0 ; j < len - 1 -i;j++) { if(p[j] > p[j+1]) { int t = p[j]; p[j] = p[j+1]; p[j+1] = t; flag = 0; } } if(flag) { break; } } //Void can also be used, because the operations are all pointer related, and the value of array a has changed. return p; }
Number of array elements 5 3 5 9 12 21
References
-
[Website] Blog Park The Difference between Array Name and Pointer in C Language
Comment
Comment area
0F C # does not include some contents of the c language, will it be used in C #? Machine code generates assembly, assembly generates C, and C generates everything.
0F Follow Big Brother C 0F Only c++'s passing meow refueling 0F 233333, your name is very 6, maoPao, a bit anti X-man X-type (face covered)