Trianglify is a javascript library that can generate colorful triangular mesh backgrounds. The js plug-in can generate very beautiful SVG background images. You can download and save the generated background images as SVG files.
Trianglify uses d3. js to generate polygons and SVG and SVG filter rendering effects. At the same time, the plug-in also uses the colorbrowser color palette to make the plug-in run faster.
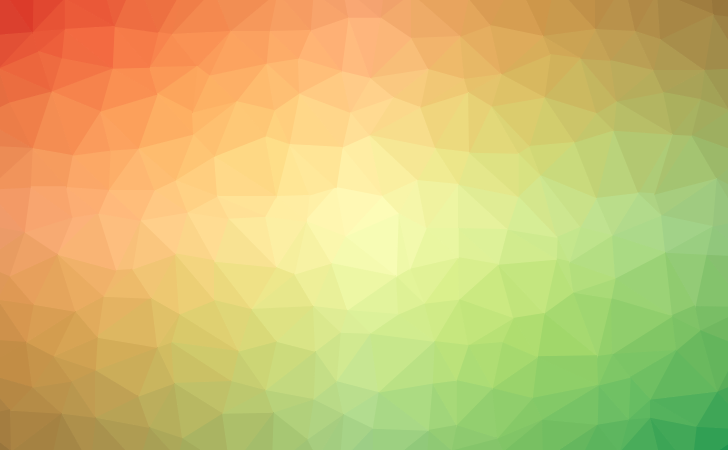
Let's take a look at the most basic example
<! DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>Examples</title> </head> <body> <script src=" https://cdnjs.cloudflare.com/ajax/libs/trianglify/0.2.0/trianglify.min.js "></script> <script> var pattern = Trianglify({ width: window.innerWidth, height: window.innerHeight }); document.body.appendChild(pattern.canvas()) </script> </body> </html>
Then look at the API and parameters:
Trianglify only exposes one method to the global command space, called Trianglify. It accepts a single option object as a parameter, and then returns a pattern object.
var Trianglify = require('trianglify'); // Only required in node.js
var pattern = Trianglify({width: 200, height: 200})
This mode object contains options and geometric data about generating patterns, as well as the implementation of rendering.
pattern.opts
Objects containing options for generating patterns
pattern.polys
The colors and fixed points of the polygons that make up the pattern are in the following format:
[
['color', [vertex, vertex, vertex]],
['color', [vertex, vertex, vertex]],
...
]
pattern.svg()
SVG rendering function. Returns the DOM node of an SVG element.
pattern.canvas([HTMLCanvasElement])
The rendering function of canvas. If no parameter is passed, a DOM node of HTMLCanvasElement is returned. If an existing canvas element is passed in as a parameter, the pattern will be rendered into the canvas.
To use it in node.js, you need to install the optional dependency node canvas
pattern.png()
PNG rendering function. Returns the URI of a base64 encoded PNG data. View and demonstrate how to decode it into a file examples/save-as-png.js
option
Trianglify is configured with an incoming option object as the only parameter. The following options are supported:
width
Integer, The default value is 600. Specifies the width of the generated pattern, in px.
height
Integer, The default value is 400. Specifies the height of the generated pattern, in px.
cell_size
Integer, The default value is 75. Specifies the size of the mesh used to generate triangles. The higher the value, the rougher it will be, and the smaller it will be, the more delicate it will be. It should be noted that a small value will significantly increase the running time of Trianglify.
variance
Decimal between 0 and 1 (including 1), default is 0.75. Specifies the random number of triangles generated.
seed
Number or string, the default value is null. Create a seed for the random number generator for duplicate pictures.
x_colors
A string or array of CSS format colors. The default value is' random '. Specifies the color gradient used on the X axis.
Valid character values are 'random' or a color name on the colorbrowser palette (such as' YlGnBu 'or' RdBu '). When set to 'random', a gradient will be randomly selected from the colorbrowser library.
Valid array values should specify color stops in any CSS format (such as ['# 000000', '# 4CAFE8', '# FFFFFF']).
y_colors
CSS format color string or array. The default value is' match_x '. When set to match_x, the same gradient is applied to the X and Y axes. Y_colors accepts the same parameter values as x_colors.
color_space
String, The default value is' lab '. Sets the color space used to generate gradients. The supported values are rgb, hsv, hsl, hsi, lab and hcl.
color_function
Specify a custom function to color the triangle, which is null by default. Accept a function that uses the x and y coordinates of the triangle center as parameters to rewrite the standard gradient. This function returns a color string in CSS format.
Here is an example of using HSL color format to generate rainbow patterns:
var colorFunc = function(x, y) {
return 'hsl('+Math.floor(Math.abs(x*y)*360)+',80%,60%)';
};
var pattern = Trianglify({color_function: colorFunc})
stroke_width
Number, The default value is 1.51. Specifies the edge width of the triangle in the pattern. The default value is an ideal value for antialiasing when rendering a pattern as canvas.
const defaultOptions = {
width: 600,
height: 400,
cellSize: 75,
variance: 0.75,
seed: null,
xColors: 'random',
yColors: 'match',
fill: true,
palette: trianglify.colorbrewer,
colorSpace: 'lab',
colorFunction: trianglify.colorFunctions.interpolateLinear(0.5),
strokeWidth: 0,
points: null}
width
Integer, default is 600. Specifies the width of the pattern to generate (in pixels).
height
Integer, default is 400. Specifies the height of the pattern to be generated (in pixels).
cellSize
Integer, 75 by default. Specifies the pixel size of the mesh used to generate triangles. The larger the value, the thicker the pattern, and the smaller the value, the finer the pattern. Note that very small values can significantly increase the running time of Trianglify.
variance
The decimal value between 0 and 1 (inclusive). The default value is 0.75. Specifies the random quantity used when generating triangles. You can set it higher than 1, but doing so may result in a pattern that includes "gaps" at the edges.
seed
String, default to null Seeds a random number generator to create repeatable patterns. When set to null, RNG uses random values from the environment as seed. An example use is to seed blog post titles to generate a unique but consistent triangulation pattern for each post on a blog site.
xColors
False、 String or CSS format color array, default is' random ' Specifies the color gradient used on the x-axis.
Valid string values are 'random' or the name of the colorbrowser palette (that is, 'YlGnBu' or 'RdBu'). When set to Random, a gradient is randomly selected from the colorbrewer library.
Valid array values should specify the color code in any CSS format (that is, ['# 000000', '# 4CAFE8', '# FFFFFF']).
yColors
False, String or CSS format color array, default is' match ' When set to Match, the x-axis color gradient is used on both axes. Otherwise, accept the same options as xColors.
palette
Random is an array of color combinations to select when using xColors or yColors. See src/utils/colorbrewer.js for the format of this data.
colorSpace
String, default is' lab ' Sets the color space used to generate gradients. The supported values are rgb, hsv, hsl, hsi, lab, and hcl. For some background information on why this is important, see this blog post.
colorFunction
Specifies the user-defined function for coloring triangles, which is null by default Accept a function that covers standard gradient shading. This function passes various data about patterns and each polygon, and must return a Chroma.js color object.
For more information about built-in color functions and how to write custom color functions, see examples/color function example. html and. src/utils/colorFunctions.js
fill
Boolean value, the default is true Specifies whether Trianglify generated polygons should be filled.
strokeWidth
Number, default is 0. Specifies the stroke width used to outline the polygon. This can be used in conjunction with fill: false to generate a network like pattern.
points
The number of points to triangulate ([x, y]) is null by default. If not specified, an array of random points will be generated to fill the space. The point must be height within the width of the coordinate space defined by and.