Su Su
two hundred and forty-four
2024-01-02
In the previous part of this series, you learned about variables. A variable can have a single value.
There can be multiple values inside an array. This makes things easier when you have to deal with multiple variables at once. You don't have to store values in new variables.
Therefore, do not declare five variables like this:
distro1=Ubuntu distro2=Fedora distro3=SUSE distro4=Arch Linux distro5=Nix
You can initialize all of them in a single array:
distros=(Ubuntu Fedora SUSE "Arch Linux" Nix)
Unlike some other programming languages, you do not use commas as array element separators.
That's very good. Let's see how to access array elements.
Access array elements in Bash
Access array elements using indexes (positions in the array). To access array elements at index N, use:
${array_name[N]}
Like most other programming languages, arrays in the Bash shell start at index 0. This means that the index of the first element is 0, the index of the second element is 1, and the index of the nth element is n-1.
So if you want to print SUSE, you will use:
echo ${distros[2]}

🚧 $ There cannot be any spaces after {or before}. You can't use it like ${array [n]}.
Access all array elements at once
Suppose you want to print all the elements of the array.
You can use echo ${array [n]} one by one, but this is really unnecessary. There is a better and simpler way:
${array[*]}
This will provide you with all array elements.
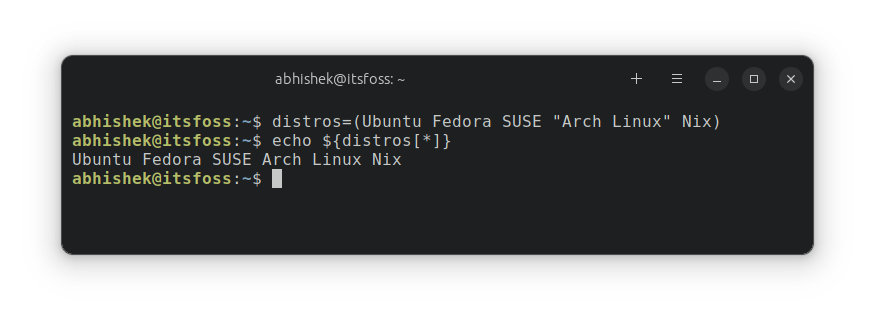
Get the array length in Bash
How do I know how many elements are in an array? There is a special method to obtain the array length in Bash:
${#array_name[@]}
It's that simple, right?
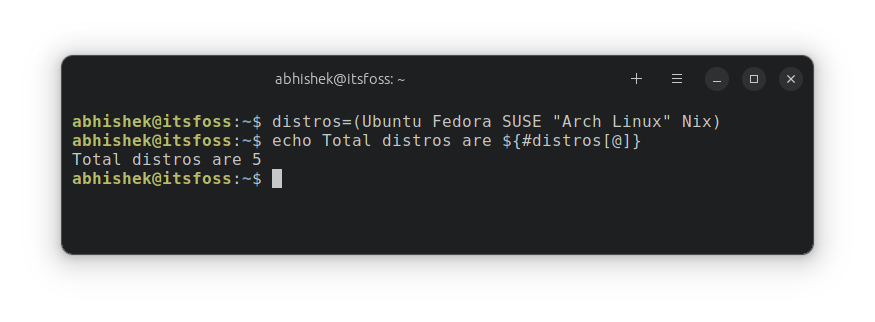
Add array elements to Bash
If you must add other elements to the array, use the+=operator to append the elements to the existing array in Bash:
array_name+=("new_value")
This is an example:

🚧 It is important to use () when appending elements.
You can also use indexes to set elements anywhere.
array_name[N]=new_value
But remember to use the correct index number. If you use it on an existing index, the new value replaces the element.
If you use the "out of bounds" index, it will still be added after the last element. For example, if the array length is 6 and you try to set a new value at index 9, the value will still be added to the seventh position (index 6) as the last element.

Delete Array Elements
You can use the shell's built-in unset to delete array elements by providing the index number:
unset array_name[N]
This is an example. I deleted the fourth element of the array.

You can also delete the entire array by unset:
unset array_name
There are no strict data type rules in Bash. You can create an array that contains both integers and strings.
Practice time
Let's practice what you have learned about Bash arrays.
Exercise 1: Create a Bash script that contains an array of five best Linux distributions. Print them all.
Now, replace the middle choice with "Hannah Montanna Linux".
Exercise 2: Create a Bash script that accepts three numbers provided by the user and prints them in reverse order.
Expected output:
Enter three numbers and press enter 12 23 44 Numbers in reverse order are: 44 23 12
I hope you enjoy learning Bash Shell scripts through this series. In the next chapter, you will learn how to use if else. Stay tuned.