Take you closer to AngularJS series:
Take you closer to AngularJS - Introduction to basic functions
Take you closer to AngularJS - experience command examples
Take you closer to AngularJS - create custom instructions
------------------------------------------------------------------------------------------------
AngularJS is a web application development framework launched by Google. It provides a series of compatible and extensible services, including data binding, DOM operation, MVC design pattern and module loading. This article focuses on the use of AngularJS instructions. Before we enter the topic, we will quickly browse the basic usage of AngularJS.
AngularJS is not just a class library, but provides a complete framework. It avoids interaction with multiple class libraries and requires you to be familiar with the tedious work of multiple sets of interfaces. It is designed by developers of Google Chrome and leads the development of next generation Web applications. Maybe we won't use AngularJS in 5 or 10 years, but its design essence will always be used.
If you know the developers of AngularJS, you will certainly be excited about the AngularJS custom instructions (its function is equivalent to the custom controls under the. NET platform). Custom commands allow you to extend HTML tags and features. Directives can be reused and used across projects.
User defined instructions have been widely used, and it is worth mentioning that- Wijmo control set 。 It contains nearly 50 AngularJS based controls. Wijmo is an HTML5 front-end control set for creating desktop and mobile Web applications. From interactive charts to powerful table controls, Wijmo contains almost everything we need. Can be accessed from Official website Learn more about Wijmo. Therefore, Wijmo is a good reference example for learning AngularJS: AngularJS Directive Gallery
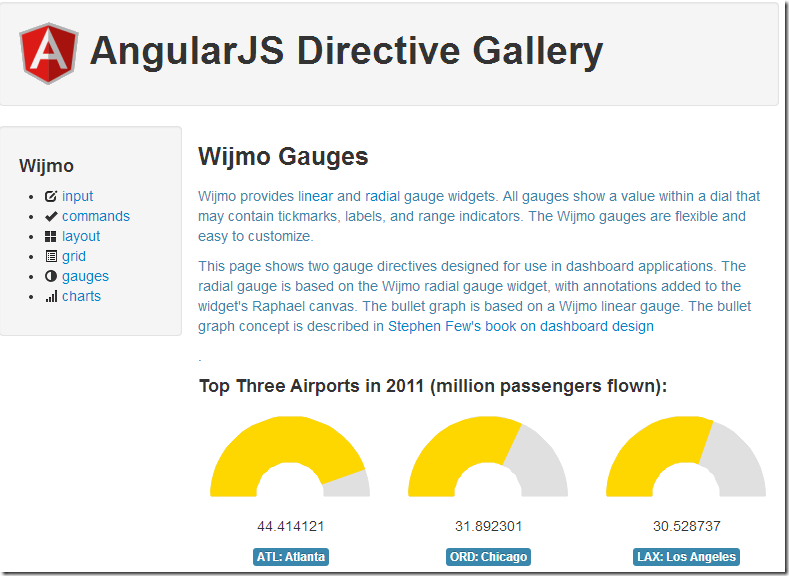
It is very easy to create custom instructions. Instructions can be tested, maintained, and reused in multiple projects.
To use AngularJS, you need to reference script files on HTML pages and add ng app features to HTML or Body tags. The following is a simple example of using AngularJS:
<html> <head> <script src=" http://code.angularjs.org/angular-1.0.1.js "></script> </head> <body ng app ng init="msg='Grapevine Controls Team Blog '"> <input ng-model="msg" /> <p>{{msg}}</p> </body> </html>
When AngularJS is loaded, it will search for the ng app feature in the document. This tag is usually set to the main module of the project. Once found, Angular will operate on the document.
In this example, the ng init feature initializes an msg variable "Grapevine Control Team Blog", and the ng model feature binds it to the input control in a two-way manner (note: braces are binding marks). AngularJS will parse this tag and update the msg text value in real time as the input value changes. You can view the effect from the link: Click to enter
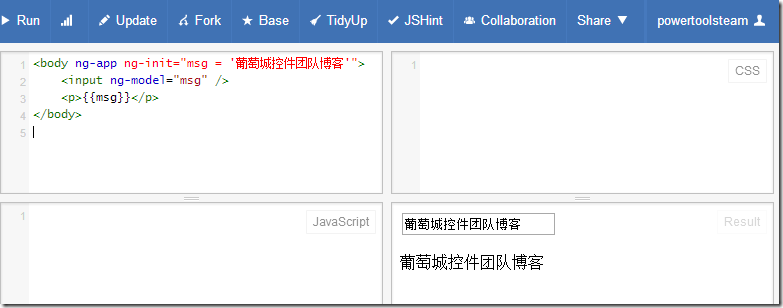
AngularJS module
Modules can be said to be the root of AngularJS. It contains configuration, control, filtering, factory mode, commands and other modules.
If you are familiar with it NET platform, but initially learn Angular. The following table is a brief comparison to help you understand the role play in Angular:
AngularJS |
.NET |
abstract |
module |
Assembly |
Application development module |
controller |
ViewModel |
Controller, starting the organizational role between different levels |
scope |
DataContext |
Provide binding data for the view |
filter |
ValueConverter |
Modify Data Before Transferring Data to View |
directive |
Component |
Reusable UI elements can also be understood as front-end plug-ins |
factory, service |
Utility classes |
Provide services for other module elements |
For example, the following code creates a module using controllers, filters, and instructions:
// the main (app) module var myApp = angular.module("myApp", []); // add a controller myApp.controller("myCtrl", function($scope) { $scope.msg = "grapecity team blog"; }); // add a filter myApp.filter("myUpperFilter", function() { return function(input) { return input.toUpperCase(); } }); // add a directive myApp.directive("myDctv", function() { return function(scope, element, attrs) { element.bind("mouseenter", function() { element.css("background", "yellow"); }); element.bind("mouseleave", function() { element.css("background", "none"); }); } });
In the above example, the first parameter of the module method is the name of the module, and the second parameter is the list of its dependent modules. We have created an independent module that is independent of other modules. So the second parameter is an empty array (Note: even if it is empty, we must fill in this parameter. Otherwise, this method will retrieve the previous module with the same name). This part will be elaborated in the following articles.
The controller constructor obtains the $scope object, which is used to store all the interfaces and methods exposed by the controller. The scope is passed to the view and instruction layers by Angular. In this example, the controller adds the msg attribute to the scope object. An application module can contain multiple controllers. Each controller performs its own duties and controls one or more views.
The filter constructor returns a method to change the way input text is displayed. Angular provides many built-in filters. At the same time, you can add custom filters in the same way as Angular built-in filters. In this example, the conversion from lowercase to uppercase is implemented. Filter can not only format text values, but also change arrays. AngularJS built-in formatting filters include number, date, currency, uppercase and lowercase. Array filters include filter, orderBy and limitTo. Filter needs to set parameters, and the syntax format is also fixed: someValue | filterName : filterParameter1 : filterParameter2... .
The directive constructor returns a method that is used to pass an element and modify it according to the parameters in the scope. In the example, we bound mouseenter and mouseleave events to switch text highlighting. This is a simple instruction, and the following chapter will show how to create some complex instructions.
The following is a page built with modules:
<body ng-app="myApp" ng-controller="myCtrl"> <input ng-model="msg" /> <p my-dctv > {{msg | myUpperFilter }} </p> </body>
You can view the effect from the link: Click to enter
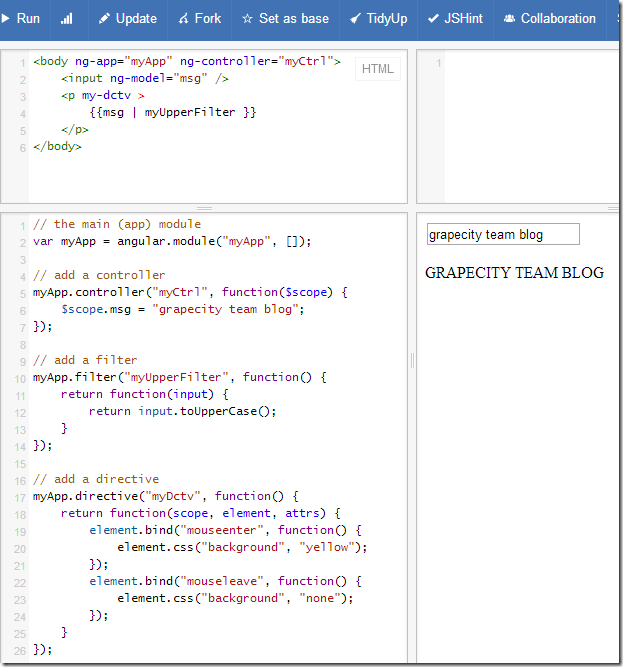
Note that module, controller and filter are used as property values. They represent JavaScript objects, so names are case sensitive. The name of the instruction is also an attribute value. It is parsed as an HTML tag, so it is case sensitive. But AngularJS will automatically convert these features to lowercase. For example, the "myDctv" instruction will become "my dctv" (just like the built-in instructions ngApp, ngController, and ngModel will be converted to "ng app", "ng controller", and "ng model").
Project organization structure
AngularJS can be used to create large Web projects. You can split a project into multiple modules and a module into multiple module files. At the same time, you can organize these files according to your usage habits.
List a typical project structure:
Root
default.html
styles
app.css
partials
home.html
product.html
store.html
scripts
app.js
controllers
productCtrl.js
storeCtrl.js
directives
gridDctv.js
chartDctv.js
filters
formatFilter.js
services
dataSvc.js
vendor
angular.js
angular.min.js
If you only want to use one module in the project, you can define it as follows:
// app.js angular.module("appModule", []);
If you want to add elements to a module, you can call the module by name to add them to it. For example: formatFilter.js The file contains the following elements:
// formatFilter.js //Get module by name var app = angular.module("appModule"); //Add a filter to the module app.filter("formatFilter", function() { return function(input, format) { return Globalize.format(input, format); } }})
If your application contains multiple modules, please add references to other modules when adding modules. For example, an application contains three modules: app, controls, and data:
//App.js (the module named app depends on the controls and data modules) angular.module("app", [ "controls", "data"]) //Controls. js (the controls module depends on the data module) angular.module("controls", [ "data" ]) //Data.js (the data module has no dependencies, and the array is empty) angular.module("data", [])
The main page of the application needs to declare the ng app directive, and AngularJS will automatically add the required references:
<html ng-app="app"> ... </html>
After the above declaration, you can use the elements declared by the other three modules in all pages.
In this article, we learned the basic usage and structure of AngularJS. In the next chapter, we will explain the basic concept of instructions, and create some examples to help you understand the role of instructions.
This article is from“ Grapevine Control Blog ”Blog, please keep this source http://powertoolsteam.blog.51cto.com/2369428/1410370