Eloquent ORM instance tutorial - ORM overview, model definition and basic query
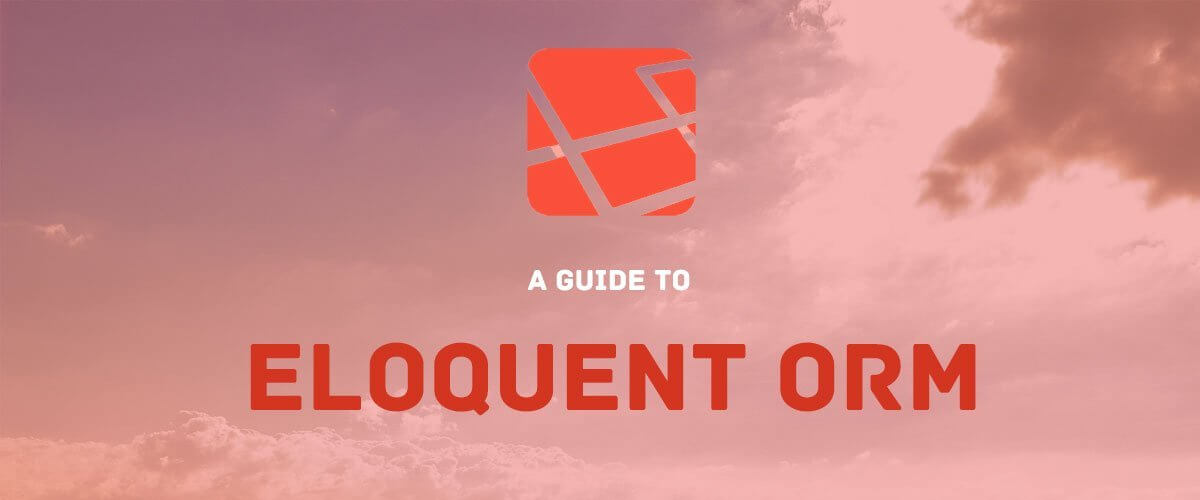
1. Primer
Before officially entering this section, let's take a look at what ORM is.
ORM, Object Relational Mapping is a mapping between relational databases and business entity objects. In this way, when we operate specific business objects, we do not need to deal with complex SQL statements, but simply operate on object properties and methods.
The two most common implementations of ORM are ActiveRecord and DataMapper. ActiveRecord is particularly popular and can be seen in many frameworks. The main difference between the two is that in ActiveRecord, the model corresponds to the data table one by one, while in DataMapper, the model and the data table are completely separated.
The Eloquent ORM in Laravel also uses the ActiveRecord implementation method. Each Eloquent model class corresponds to a table in the database. We can add, delete, and query the database by calling the corresponding method of the model class.
2. Define model
2.1 Create model
We use Artisan commands make:model
Generate model class, which is located in app
Directory, we can also specify the generation directory when creating:
php artisan make:model Models/Post
This will app
Generate a Models
Directory, and in the Models
Generate a Post
Model class. All model classes in Larave inherit from Illuminate\Database\Eloquent\Model
Class.
2.2 Specify Table Name
If not specified manually, the default is Post
The corresponding data table is posts
, and so on. You can also set $table
Attribute custom table name:
public $table = 'posts';
2.3 Specify Primary Key
Eloquent default data table primary key is id
, of course, you can also set $primaryKey
Attribute user-defined primary key:
public $primaryKey = 'id';
2.4 Time stamp setting
By default, the Eloquent model class automatically manages timestamp columns create_at
and update_at
(If these two columns are set when defining migration). If you want to cancel automatic management, you can set $timestamps
Property is false
:
public $timestamps = false;
Also, if you want to set the format of the timestamp, you can use $dateFormat
Attribute, which determines the format in which the date and time are stored in the database and displayed:
//Set the date time format to Unix timestamp protected $dateFormat = 'U';
For more information about date time format settings, please refer to Format part of php official function date 。
3. Query Data
3.1 Obtaining Multiple Models
We can use the all
Method to obtain all model instances. For example, we can obtain all articles through the following methods:
<? php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Http\Requests; use App\Http\Controllers\Controller; use App\Models\Post; class TestController extends Controller { /** * Display a listing of the resource. * * @return Response */ public function index() { //Get multiple Eloquent models $posts = Post::all(); dd($posts); } }
The corresponding output result is:
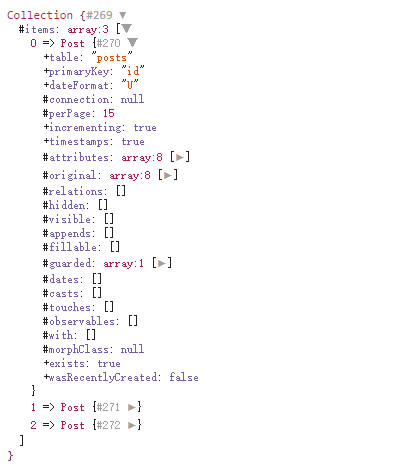
The visible output result is a collection of model arrays $items
Element corresponds to one Post
Model instance.
In addition, we need to understand that every Eloquent model is a query builder itself, so we can call methods on all query builders, but the first method call should use static method calls:
$posts = Post::where('id','<',3)->orderBy('id','desc')->take(1)->get(); dd($posts);
The corresponding output result is:
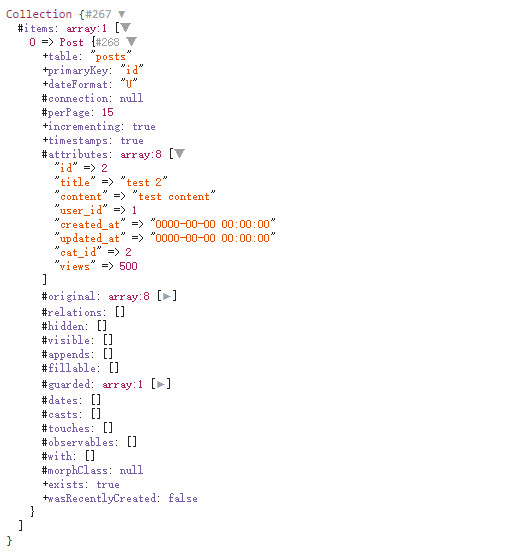
You may have noticed that the returned results of model query are Illuminate\Database\Eloquent\Collection
This class implements the ArrayAccess
Interface, so we can access this instance just like an array. In addition, the Collection class also provides many other useful methods to process the query results. See the source code for details.
Since Eloquent model is a query builder, naturally it also supports data acquisition by grouping blocks:
Post::chunk(2,function($posts){ foreach ($posts as $post) { echo $post->title.'
'; } });
The output results are as follows:
test 1 test 2 test 3
3.2 Obtaining a single model
You can use the query builder method to obtain a single model instance:
$post = Post::where('id',1)->first(); dd($post);
Of course, you can also use the shortcut provided by the Eloquent model class find
:
$post = Post::find(1);
Both outputs are the same:
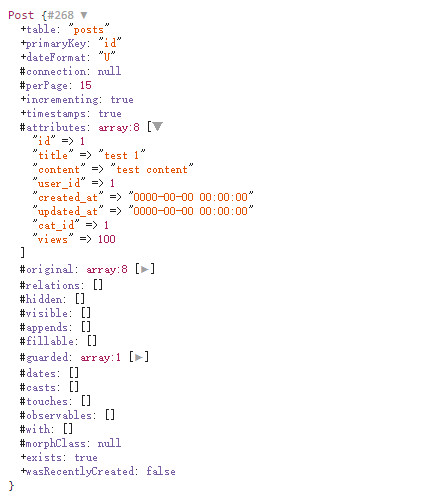
If no corresponding table record is found, it will output null
If we want to catch and process the exception with null query results, for example, jump to page 404, we can use findOrFail
perhaps firstOrFail
Method, if the table record exists, both return the first record obtained, otherwise throw Illuminate\Database\Eloquent\ModelNotFoundException
Exception.
3.3 Aggregate Function Query
If you want to count, count, max/min, average and other aggregation operations on the query results, you can use the corresponding methods on the query builder. We query the total number of articles:
$count = Post::where('id','>',0)->count(); echo $count;
The output result is three
, or we want to get the maximum number of articles read:
$views = Post::where('id','>',0)->max('views'); echo $views;
The output result is eight hundred
。