Simple task management system
Introduction: Laravel has finally launched the 5.1 version of the Quick Start Guide. After reading the discussion on reddit, Mr. Xueyuan learned about the news and immediately started to translate it, hoping to help Laravel learners.
1. Introduction
The quick start guide will give a basic introduction to the Laravel framework, including database migration, Eloquent ORM, routing, verification, views, blade templates, and so on. If you are a Larravel novice or even unfamiliar with PHP frameworks before, this will be a good starting point for you. If you have used Larravel to get other PHP frameworks, you can consider jumping to the Advanced Guide (in translation).
To demonstrate the basic use of the Larravel feature, we will build a simple To Do List to track all tasks to be completed. The complete code of this tutorial has been published on Github: https://github.com/laravel/quickstart-basic 。
2. Installation
Of course, the first thing you need to do before starting is to install a new Larravel application. You can use Homestead virtual machine Or run the application in the local PHP development environment. After setting the development environment, you can use the Composer command below to install applications:
composer create-project laravel/laravel quickstart --prefer-dist
Of course, you can also install it locally by cloning the GitHub repository:
git clone https://github.com/laravel/quickstart-basic quickstart cd quickstart composer install php artisan migrate
If you don't know how to build a local development environment, please refer to Homestead and install file.
3. Prepare database
Database migration
First, let's use migration to define the data table for processing all tasks. Larave's database migration feature provides a simple way to define and modify the data table structure: instead of letting each member of the team add columns to the local database, simply run the migration you submit to source control to create and modify the data table.
So, let's create a data table that processes all tasks. Artisan Command It can be used to generate multiple classes to save repetitive work. In this example, we use make:migration
Command generation tasks
Corresponding data table migration:
php artisan make:migration create_tasks_table --create=tasks
The migration file generated by this command is located in the database/migrations
Directory, you may have noticed that, make:migration
The command has added the self increasing ID and timestamp to the migration file. Next, we need to edit the file to add more columns to the data table tasks
:
<? php use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class CreateTasksTable extends Migration{ /** * Run the migrations. * * @return void */ public function up() { Schema::create('tasks', function (Blueprint $table) { $table->increments('id'); $table->string('name'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::drop('tasks'); } }
To run the migration, you can use the Artisan command migrate
。 If you use Homestead, you should run this command in the virtual machine:
php artisan migrate
This command will create all the data tables defined in the migration file for us. If you use the database client software to view the database, you can see that a new one has been created tasks
Table, which contains the columns we defined in the migration. Next, we are going to define an Eloquent ORM model for this data table.
Eloquent model
The default ORM used by Laravel is Eloquent, Eloquent Using models makes data access simple and easy. Generally, each Eloquent model has a corresponding data table.
So we need to define a tasks
Table corresponding Task
Model, we also use Artisan command to generate this model:
php artisan make:model Task
The model class is located in app
Directory. By default, the model class is empty. We don't need to tell which data table the Eloquent model corresponds to Eloquent Document As mentioned in, the default data table here is tasks
, the following is the empty model class:
<? php namespace App; use Illuminate\Database\Eloquent\Model; class Task extends Model{ // }
For more details on Eloquent model classes, see the complete Eloquent Document 。
4. Routing
Create Route
Next, we need to define some routes for applications. Routes are used to match the page URL to the controller or anonymous function to be executed when a user accesses a specified page. By default, all Larave routes are defined in app/Http/routes.php
。
In this application, we need at least three routes: show routes for all tasks, add routes for new tasks, and delete routes for existing tasks. Next, let's app/Http/routes.php
Create these three routes in the file:
<? php use App\Task; use Illuminate\Http\Request; /** * Display All Tasks */ Route::get('/', function () { // }); /** * Add A New Task */ Route::post('/task', function (Request $request) { // }); /** * Delete An Existing Task */ Route::delete('/task/{id}', function ($id) { // });
Show View
Next, let's fill in /
Route. In this route, we want to render an HTML template, which contains the form for adding new tasks and displaying the task list.
In Larravel, all HTML templates are stored in resources/views
Directory, we can use view
The function returns one of the templates from the route:
Route::get('/', function () { return view('tasks'); });
Of course, next we need to create this view.
5. Create Layout&View
This application contains only one view for simple processing, including the form for adding new tasks and the list of all tasks. In order to give you an intuitive visual effect, we post a screenshot of the view. We can see that we use the basic Bootstrap CSS style in the view:
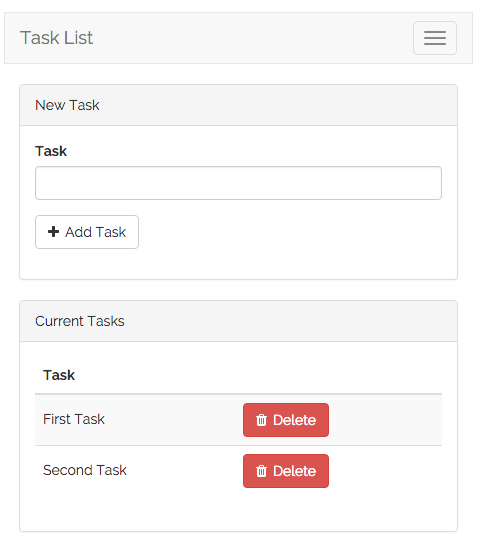
Define Layout
Almost all web applications share the same layout in different pages. For example, this application has a navigation bar at the top of the view, which will appear on each page. Larave makes it easy to share these common features by using the Blade layout in each page.
As we discussed earlier, all Larravel views are stored in resources/views
So, we are resources/views/layouts/app.blade.php
Define a new layout view in the, .blade.php
Extension indicates the use of the framework Blade template engine To render the view, of course, you can use the native PHP template. However, the tag syntax provided by Blade can help us write a cleaner and shorter template.
edit app.blade.php
The contents are as follows:
// resources/views/layouts/app.blade.php <! DOCTYPE html><html lang="en"> <head> <title>Laravel Quickstart - Basic</title> <!-- CSS And JavaScript --> </head> <body> <div class="container"> <nav class="navbar navbar-default"> <!-- Navbar Contents --> </nav> </div> @yield('content') </body> </html>
Pay attention to the @yield('content')
Part. This is a Blade instruction that specifies that the sub page of the inherited layout can inject its own content here. Next, let's define a sub view that uses this layout to provide the main content.
Define Subviews
Well, we have created the layout view of the application. Next, we need to define a view containing the form for creating new tasks and the list of existing tasks. The view file is stored in resources/views/tasks.blade.php
。
We will skip the template file of Bootstrap CSS and focus only on our concerns. Don't forget, you can select GitHub Download all resources of this app:
// resources/views/tasks.blade.php @extends('layouts.app') @section('content') <!-- Bootstrap Boilerplate... --> <div class="panel-body"> <!-- Display Validation Errors --> @include('common.errors') <!-- New Task Form --> <form action="/task" method="POST" class="form-horizontal"> {{ csrf_field() }} <!-- Task Name --> <div class="form-group"> <label for="task" class="col-sm-3 control-label">Task</label> <div class="col-sm-6"> <input type="text" name="name" id="task-name" class="form-control"> </div> </div> <!-- Add Task Button --> <div class="form-group"> <div class="col-sm-offset-3 col-sm-6"> <button type="submit" class="btn btn-default"> <i class="fa fa-plus"></i> Add Task </button> </div> </div> </form> </div> <!-- TODO: Current Tasks --> @endsection
Before continuing, let's briefly talk about this template. First, we use @extends
The command tells the Blade to use the resources/views/layouts/app.blade.php
Layout of, all @section('content')
and @endsection
The content between will be injected into app.blade.php
Laid out @yield('contents')
Command position.
Now that we have defined the basic layout and view for the application, we are ready to add code to POST /task
The path is used to handle adding new tasks to the database.
Note: @include('common.errors')
The command will load resources/views/common/errors.blade.php
We haven't defined the content in the template yet, but we will soon!
6. Add Task
Validate form input
Now that we have defined the form in the view, we need to write code to process the form request. We need to verify the form input before we can create a new task.
For this form, we will name
The field is set as required and cannot exceed 255 characters in length. If the form verification fails, it will jump to the previous page and save the error information to the one-time session Medium:
Route::post('/task', function (Request $request) { $validator = Validator::make($request->all(), [ 'name' => 'required|max:255', ]); if ($validator->fails()) { return redirect('/') ->withInput() ->withErrors($validator); } // Create The Task... });
$errors variable
Let's stop and discuss ->withErrors($validator)
part, ->withErrors($validator)
The verification error information will be stored in a one-time session so that you can pass the $errors
Variable access.
We used @include('common.errors')
Command to render the form validation error message, common.errors
Allows us to display error messages in a uniform format on all pages. We define common.errors
The contents are as follows:
// resources/views/common/errors.blade.php @if (count($errors) > 0) <!-- Form Error List --> <div class="alert alert-danger"> <strong>Whoops! Something went wrong!</strong> <br><br> <ul> @foreach ($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> </div> @endif
Note: $errors
The variable can be accessed in every Larravel view. If there is no error message, it is empty ViewErrorBag
example.
Create Task
Now that the input verification has been completed, we will formally start to create a new task. Once the new task is created successfully, the page will jump to /
。 To create a task, you can use the save
method:
Route::post('/task', function (Request $request) { $validator = Validator::make($request->all(), [ 'name' => 'required|max:255', ]); if ($validator->fails()) { return redirect('/') ->withInput() ->withErrors($validator); } $task = new Task; $task->name = $request->name; $task->save(); return redirect('/'); });
Well, here we are, we can successfully create tasks. Next, we continue to add code to the view to display all task lists.
Show existing tasks
First, we need to edit /
Route all existing tasks to the view. view
The function receives an array as the second parameter. We can pass the data to the view through the array:
Route::get('/', function () { $tasks = Task::orderBy('created_at', 'asc')->get(); return view('tasks', [ 'tasks' => $tasks ]); });
After the data is transferred to the view, we can tasks.blade.php
All tasks are displayed as a table in. Used in Blade @foreach
Processing circular data:
@extends('layouts.app') @section('content') <!-- Create Task Form... --> <!-- Current Tasks --> @if (count($tasks) > 0) <div class="panel panel-default"> <div class="panel-heading"> Current Tasks </div> <div class="panel-body"> <table class="table table-striped task-table"> <!-- Table Headings --> <thead> <th>Task</th> <th> </ th> </thead> <!-- Table Body --> <tbody> @foreach ($tasks as $task) <tr> <!-- Task Name --> <td class="table-text"> <div>{{ $task->name }}</div> </td> <td> <!-- TODO: Delete Button --> </td> </tr> @endforeach </tbody> </table> </div> </div> @endif @endsection
So far, this application is basically completed. However, when the task is completed, we have no way to delete the task. Next, we will deal with this matter.
7. Delete Task
Add Delete Button
We are tasks.blade.php
A "TODO" note is left in the view to place the delete button. When the delete button is clicked, DELETE /task
The request is sent to the application background:
<tr> <!-- Task Name --> <td class="table-text"> <div>{{ $task->name }}</div> </td> <!-- Delete Button --> <td> <form action="/task/{{ $task->id }}" method="POST"> {{ csrf_field() }} {{ method_field('DELETE') }} <button>Delete Task</button> </form> </td> </tr>
About method forgery
Although the route we use is Route::delete
, but the request method we use in the delete button form is POST
, HTML forms only support GET
and POST
Two request methods, so we need to use some way to forge DELETE
Request.
We can output method_field('DELETE')
To forge DELETE
Request, this function generates a hidden form input box, and then Laravel recognizes the input and uses its value to override the actual HTTP request method. The generated input box is as follows:
<input type="hidden" name="_method" value="DELETE">
Delete Task
Finally, let's add business logic to the route to perform the deletion operation. We can use Eloquent's findOrFail
Method Get the model instance from the database by ID, and throw 404 exceptions if it does not exist. After obtaining the model, we use the delete
Method to delete the corresponding record of the model in the database. After the record is deleted, go to /
Page:
Route::delete('/task/{id}', function ($id) { Task::findOrFail($id)->delete(); return redirect('/'); });
Read on: Advanced Guide for Novice -- Task Management System with User Function